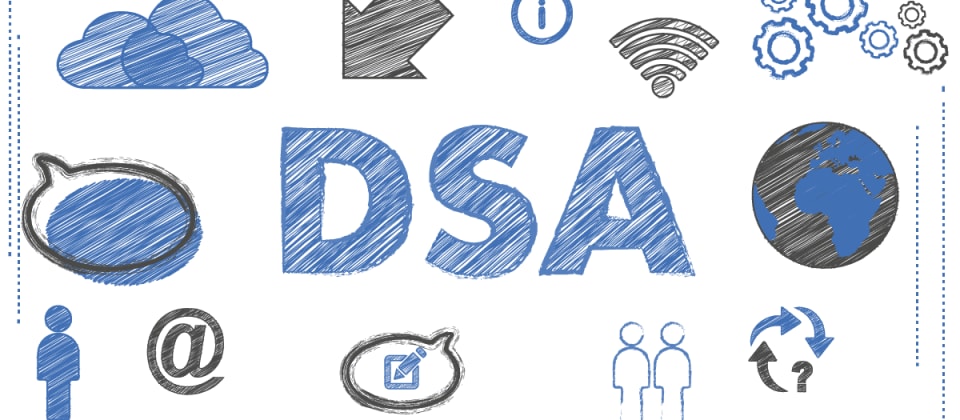
Kickstarting Your DSA Journey: A Beginner's Roadmap
Data Structures and Algorithms (DSA) are the backbone of computer science and play a crucial role in solving complex problems efficiently. Whether you're preparing for coding interviews, competitive programming, or simply honing your problem-solving skills, starting your DSA journey can feel daunting. This guide will help you take the first steps in learning DSA systematically and effectively.
Step 1: Understand Why DSA Matters
Before diving into DSA, it's essential to understand its significance. DSA helps in:
- Optimizing code for better performance.
- Solving real-world problems effectively.
- Preparing for coding interviews where DSA concepts are heavily tested.
- Building a strong foundation for advanced computer science topics like machine learning or system design.
This awareness will keep you motivated throughout your learning journey.
Step 2: Strengthen Your Programming Basics
DSA requires a solid understanding of at least one programming language. Popular choices include:
-
Python (easy to learn and has rich libraries).
-
C++ (preferred for competitive programming due to its speed).
-
Java (widely used in interviews and development).
Focus on mastering basic programming constructs like:
-
Variables and data types.
-
Loops and conditionals.
-
Functions and recursion.
-
Arrays and strings.
If you're not confident in these areas, spend time practicing simple coding problems on platforms like HackerRank
or CodeChef
.
Step 3: Learn the Fundamentals of DSA
Start with the foundational data structures and algorithms. Follow a structured order to avoid overwhelm:
Basic Data Structures
-
Arrays
-
Strings
-
Linked Lists
-
Stacks
-
Queues
Basic Algorithms
-
Searching (e.g., Linear Search, Binary Search)
-
Sorting (e.g., Bubble Sort, Merge Sort, Quick Sort)
-
Recursion basics
Use resources like YouTube tutorials or online courses to grasp these topics conceptually before moving to advanced ones.
Step 4: Practice Regularly
DSA is all about practice. Apply the concepts you learn by solving problems on coding platforms such as:
-
LeetCode
-
GeeksforGeeks
-
Codeforces
Start with easy problems, and gradually move to medium and hard problems. Aim to solve at least one problem per day to build consistency.
Step 5: Explore Advanced Topics
Once you're comfortable with the basics, move on to advanced data structures and algorithms, including:
-
Trees and Binary Search Trees
-
Heaps
-
Hashing
-
Graphs
-
Dynamic Programming
These topics are crucial for solving complex problems and performing well in coding interviews.
Step 6: Use the Right Resources
Learning DSA becomes easier with the right resources. Here are some recommendations:
-
Books: "Introduction to Algorithms" by Cormen (CLRS), "Data Structures and Algorithm Analysis" by Mark Allen Weiss.
-
Courses: Coursera, Udemy, or Coding Ninjas.
-
YouTube Channels: FreeCodeCamp, CodeWithHarry, Abdul Bari.
Step 7: Participate in Coding Challenges
Join coding competitions on platforms like Codeforces
, CodeChef
, and HackerRank
. These contests will test your knowledge under time constraints and help you think critically.
Step 8: Review and Revise
Revisiting solved problems and key concepts is crucial. Maintain a notebook or digital document of:
-
Common algorithms.
-
Problem-solving strategies.
-
Mistakes made and lessons learned.
Periodic revision solidifies your understanding and prepares you for future challenges.
Step 9: Stay Patient and Persistent
Learning DSA is a marathon, not a sprint. There will be moments of frustration, but persistence is key. Remember that every problem you solve brings you closer to mastery.
Starting with DSA may seem overwhelming, but a structured approach, consistent practice, and the right resources will make your journey rewarding. Keep challenging yourself, and don't hesitate to seek help from the programming community when needed. With time and effort, you'll become proficient in DSA and ready to tackle any coding challenge that comes your way!
Happy Coding!
5 Reactions
0 Bookmarks