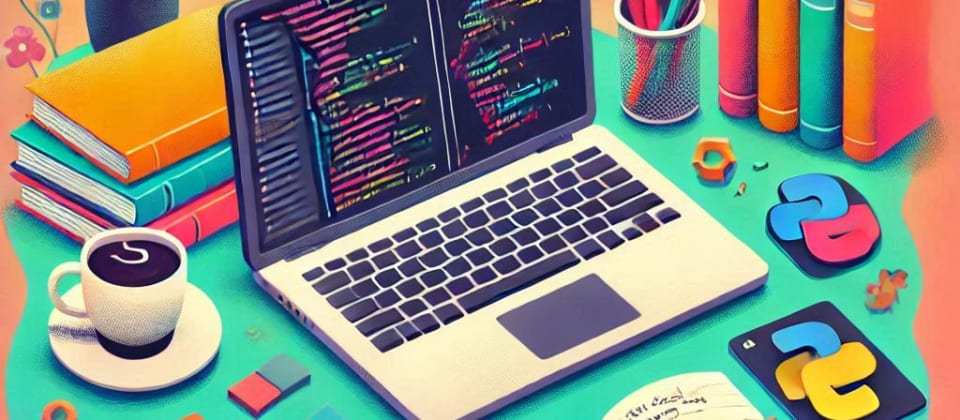
NumPy : To The Point
Introduction to NumPy: The Backbone of Numerical Computing in Python
In the world of data science, machine learning, and scientific computing, NumPy stands as one of the most fundamental and essential libraries in Python. NumPy, short for Numerical Python, provides a powerful N-dimensional array object and a collection of routines for fast operations on arrays, including mathematical, logical, shape manipulation, sorting, selecting, and more.
Why NumPy?
-
Efficient: NumPy is highly efficient in handling large amounts of data, providing functionalities that perform computations faster than native Python code.
-
Array Operations: It allows you to perform element-wise operations on arrays, similar to matrix operations.
-
Integration: Integrates well with other libraries like SciPy, Pandas, and Matplotlib, making it versatile for various types of data manipulation and visualization tasks.
Getting Started with NumPy
First, you need to install NumPy. You can install it using pip:
pip install numpy
Once installed,you can import it into your python script or Jupyter notebook:
import numpy as np
NumPy Array
The core of NumPy is the ndarray(N-dimension array) object.Unlike Python list, NumPy arrrays are homogenius,meaning all elements have to be of the same type. This homogeneity allows NumPy to efficiently perform operations over array.
Creating Array
you can create arrays in multiple ways:
1.From Lists:
import numpy as np
my_list= [1,2,3,4,5]
my_array=np.array(my_list)
print(my_array)
Output : [1 2 3 4 5 ]
2.Using built-in function:
zeros_array = np.zeros(5) #Create an array of zeros
ones_array=np.ones((2,3)) #Create a 2*3 array of ones
arange_array = np.arrange(10) #Create an array with values from 0 to 9
3.Random Array:
random_array= np.random.random(3,3) #create a 3*3 array with random value
Array Attributes
NumPy arrays come with several attributes that help you understand the array:
array = np.array([[1, 2, 3], [4, 5, 6]])
print("Shape:", array.shape) # (2, 3)
print("Data Type:", array.dtype) # int64 (or int32 depending on your system)
print("Size:", array.size) # 6
print("Number of Dimensions:", array.ndim) # 2
Array Operations
NumPy allows various operations on arrays. Here are some essential ones:
Element-wise Operations
You can perform arithmetic operations on arrays just like you would with scalars:
a = np.array([1, 2, 3])
b = np.array([4, 5, 6])
print("Addition:", a + b) # [5 7 9]
print("Subtraction:", a - b) # [-3 -3 -3]
print("Multiplication:", a * b) # [4 10 18]
print("Division:", a / b) # [0.25 0.4 0.5]
Aggregate Functions
NumPy provides several functions to perform statistical operations on arrays:
array = np.array([1, 2, 3, 4, 5])
print("Mean:", np.mean(array)) # 3.0
print("Sum:", np.sum(array)) # 15
print("Standard Deviation:", np.std(array)) # 1.4142135623730951
print("Maximum:", np.max(array)) # 5
print("Minimum:", np.min(array)) # 1
Matrix Operations
NumPy supports matrix operations, which are crucial in data science and machine learning:
matrix1 = np.array([[1, 2], [3, 4]])
matrix2 = np.array([[5, 6], [7, 8]])
print("Matrix Multiplication:\n", np.dot(matrix1, matrix2))
Output:
[[19 22]
[43 50]]
Reshaping and Broadcasting
Reshaping arrays allows you to change their dimensions without altering the data:
array = np.arange(12)
reshaped_array = array.reshape((3, 4))
print(reshaped_array)
Output:
[[ 0 1 2 3]
[ 4 5 6 7]
[ 8 9 10 11]]
Broadcasting lets you perform operations on arrays of different shapes:
array = np.array([1, 2, 3])
scalar = 2
print("Broadcasted Addition:", array + scalar) # [3 4 5]
Advanced feature
Fancy Indexing and Slicing:
NumPy provides powerful indexing and slicing capabilities to access subsets of data:
array = np.array([1, 2, 3, 4, 5])
# Slicing
print("Slice:", array[1:4]) # [2 3 4]
# Fancy Indexing
indices = [0, 2, 4]
print("Fancy Indexing:", array[indices]) # [1 3 5]
Boolean Masking
You can use boolean conditions to filter data:
array = np.array([1, 2, 3, 4, 5])
mask = array > 2
print("Boolean Masking:", array[mask]) # [3 4 5]
Linear Algebra Functions
NumPy includes a submodule dedicated to linear algebra operations:
from numpy.linalg import inv, eig
matrix = np.array([[1, 2], [3, 4]])
inverse = inv(matrix)
eigenvalues, eigenvectors = eig(matrix)
print("Inverse:\n", inverse)
print("Eigenvalues:\n", eigenvalues)
print("Eigenvectors:\n", eigenvectors)
Whether you're handling large datasets, performing complex mathematical operations, or simply want faster computations, NumPy is the go-to library.
Investing time in mastering NumPy will undoubtedly pay off as you dive deeper into the realms of data analysis and machine learning. Its robust features and versatility will enhance your productivity and open up new possibilities for your projects.
Thanks for reading ~ Ujjwal Paliwal
5 Reactions
1 Bookmarks