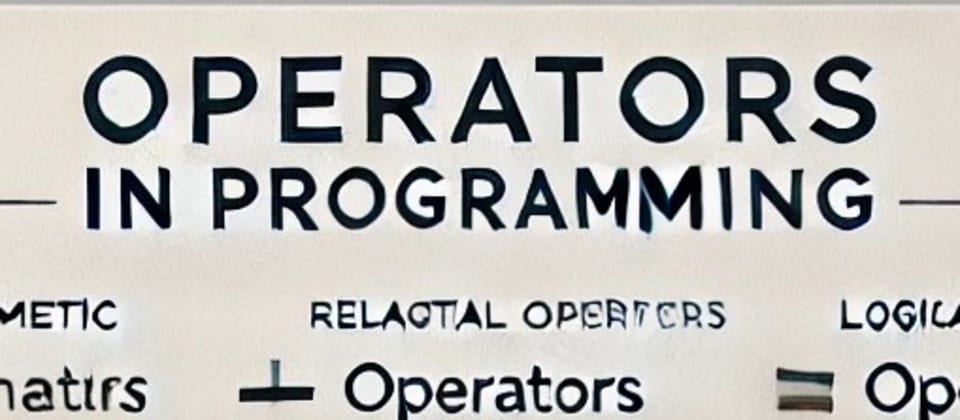
Operators(Types of operators...)
Hey Coders! Today, we are going to dive deeper into the concept of operators in programming. We'll explore the various types of operators. These include arithmetic operators for basic calculations, relational operators for comparisons, logical operators for boolean logic, assignment operators for value assignments, bitwise operators for binary manipulations, unary operators for single operand operations, the ternary operator for concise conditionals, and special operators like sizeof and type casting."
List of content:
- Defintion.
- Need of Operators.
- Types of Operators.
Let's start.....
Definition:
Operators in programming are symbols or keywords that perform specific operations on data. They are fundamental to any programming language and are used to manipulate variables and values.
Need of Operators:
They provide a way to perform a wide range of operations, from simple arithmetic calculations to complex logical decisions. Without operators, it would be nearly impossible to perform fundamental programming tasks such as:
- Mathematical Calculations: Arithmetic operators allow you to perform basic math operations, which are crucial for tasks ranging from simple addition to complex algorithms.
- Decision Making: Relational and logical operators enable the comparison of values and implementation of decision-making logic, making it possible to write conditional statements.
- Variable Management: Assignment operators facilitate the storage and updating of values in variables, essential for maintaining and manipulating data.
- Bitwise Operations: Bitwise operators allow for low-level data processing, which is important in systems programming and performance-critical applications.
- Efficiency and Readability: Operators help make code more concise and readable, reducing the complexity and improving maintainability of programs.
Types of Operators:
- Arithmetic Operators.
- Relational Operators.
- Logical Operators.
- Bitwise Operators.
- Assignment Operators.
- Other Operators.
1. Arithmetic Operators:
Arithmetic operators are used to perform basic mathematical operations. The most common arithmetic operators include:
- Addition (+): Adds two operands.
- Subtraction (-): Subtracts the second operand from the first.
- Multiplication (*): Multiplies two operands.
- Division (/): Divides the first operand by the second.
- Modulus (%): Returns the remainder of the division of the first operand by the second.
Operator | Description | Syntax | Example | Result |
---|---|---|---|---|
+ |
Addition | operand1 + operand2 |
5 + 3 |
8 |
- |
Subtraction | operand1 - operand2 |
10 - 4 |
6 |
* |
Multiplication | operand1 * operand2 |
6 * 7 |
42 |
/ |
Division | operand1 / operand2 |
20 / 4 |
5 |
% |
Modulus | operand1 % operand2 |
22 % 7 |
1 |
++ |
Increment | operand++ or ++operand |
a = 5; a++; |
6 |
-- |
Decrement | operand-- or --operand |
a = 5; a--; |
4 |
+ |
Unary Plus | +operand |
+5 |
5 |
- |
Unary Minus | -operand |
-5 |
-5 |
Examples:
1. Addition:
a = 5
b = 3
result = a + b
print(result) # Output: 8
2. Subtraction:
a = 10
b = 4
result = a - b
print(result) # Output: 6
3. Multiplication:
a = 6
b = 7
result = a * b
print(result) # Output: 42
4. Division:
a = 20
b = 4
result = a / b
print(result) # Output: 5.0
5. Modulus:
a = 22
b = 7
result = a % b
print(result) # Output: 1
6. Increment:
a = 5
a++
print(a) # Output: 6
7. Decrement:
a = 5
a--
print(a) # Output: 4
8. Unary Plus:
a = -5
result = +a
print(result) # Output: -5
9. Unary Minus:
a = 5
result = -a
print(result) # Output: -5
2. Relational Operators:
Relational operators are used to compare two values or expressions. These comparisons typically yield a boolean result, either true or false. Here are the common relational operators:
- Equal to (==): Checks if two operands are equal.
- Not Equal to (!=): Checks if two operands are not equal.
- Greater than (>): Checks if the left operand is greater than the right operand.
- Less than (<): Checks if the left operand is less than the right operand.
- Greater than or Equal to (>=): Checks if the left operand is greater than or equal to the right operand.
- Less than or Equal to (<=): Checks if the left operand is less than or equal to the right operand.
Operator | Description | Syntax | Example | Result |
---|---|---|---|---|
== |
Equal to | operand1 == operand2 |
5 == 5 |
true |
!= |
Not Equal to | operand1 != operand2 |
5 != 3 |
true |
> |
Greater than | operand1 > operand2 |
10 > 5 |
true |
< |
Less than | operand1 < operand2 |
5 < 10 |
true |
>= |
Greater than or Equal to | operand1 >= operand2 |
5 >= 5 |
true |
<= |
Less than or Equal to | operand1 <= operand2 |
5 <= 10 |
true |
Examples:
1. Equal to:
a = 5
b = 5
result = (a == b)
print(result) # Output: True
2. Not Equal to:
a = 5
b = 3
result = (a != b)
print(result) # Output: True
3. Greater than:
a = 10
b = 5
result = (a > b)
print(result) # Output: True
4. Less than:
a = 5
b = 10
result = (a < b)
print(result) # Output: True
5. Greater than or Equal to:
a = 5
b = 5
result = (a >= b)
print(result) # Output: True
6. Less than or Equal to:
a = 5
b = 10
result = (a <= b)
print(result) # Output: True
3. Logical Operators:
Logical operators are used to perform logical operations on boolean values (true or false). They are essential for making decisions based on multiple conditions. Here are the common logical operators:
- AND (&&): Returns true if both operands are true.
- OR (||): Returns true if at least one of the operands is true.
- NOT (!): Inverts the boolean value of the operand.
Operator | Description | Syntax | Example | Result |
---|---|---|---|---|
&& |
AND | operand1 && operand2 |
(true && false) |
false |
|| |
OR | operand1 || operand2 |
(true || false) |
true |
! |
NOT | !operand |
!true |
false |
Examples:
1. AND (&&):
a = True
b = False
result = a && b
print(result) # Output: False
2. OR (||):
a = True
b = False
result = a || b
print(result) # Output: True
3. NOT (!):
a = True
result = !a
print(result) # Output: False
4. Bitwise Operators:
Bitwise operators are used to perform operations on individual bits of binary numbers. These operators are essential for low-level programming, such as manipulating hardware registers, optimizing performance, and implementing efficient algorithms. Here are the common bitwise operators:
- AND (&): Performs a bitwise AND operation.
- OR (|): Performs a bitwise OR operation.
- XOR (^): Performs a bitwise XOR (exclusive OR) operation.
- **NOT (~): Performs a bitwise NOT (complement) operation.
- Left Shift (<<): Shifts bits to the left.
- Right Shift (>>): Shifts bits to the right.
Operator | Description | Syntax | Example | Result |
---|---|---|---|---|
& |
AND | operand1 & operand2 |
5 & 3 |
1 |
| |
OR | operand1 | operand2 |
5 | 3 |
7 |
^ |
XOR | operand1 ^ operand2 |
5 ^ 3 |
6 |
~ |
NOT | ~operand |
~5 |
-6 |
<< |
Left Shift | operand << n |
5 << 1 |
10 |
>> |
Right Shift | operand >> n |
5 >> 1 |
2 |
Examples:
1. AND (&):
a = 5 # Binary: 0101
b = 3 # Binary: 0011
result = a & b
print(result) # Output: 1 (Binary: 0001)
2. OR (|):
a = 5 # Binary: 0101
b = 3 # Binary: 0011
result = a | b
print(result) # Output: 7 (Binary: 0111)
3. XOR (^):
a = 5 # Binary: 0101
b = 3 # Binary: 0011
result = a ^ b
print(result) # Output: 6 (Binary: 0110)
4. NOT (~):
a = 5 # Binary: 0101
result = ~a
print(result) # Output: -6 (Binary: 1010 in two's complement)
5. Left Shift (<<):
a = 5 # Binary: 0101
result = a << 1
print(result) # Output: 10 (Binary: 1010)
6. Right Shift (>>):
a = 5 # Binary: 0101
result = a >> 1
print(result) # Output: 2 (Binary: 0010)
5. Assignment operators:
Assignment operators are used to assign values to variables. Along with the basic assignment operator (=), there are compound assignment operators that combine arithmetic, bitwise, and shift operations with assignment. Here are the updated common assignment operators:
- Assignment (=): Assigns the value of the right operand to the left operand.
- Add and Assign (+=): Adds the right operand to the left operand and assigns the result to the left operand.
- Subtract and Assign (-=): Subtracts the right operand from the left operand and assigns the result to the left operand.
- Multiply and Assign (*=): Multiplies the left operand by the right operand and assigns the result to the left operand.
- Divide and Assign (/=): Divides the left operand by the right operand and assigns the result to the left operand.
- Modulus and Assign (%=): Takes the modulus of the left operand by the right operand and assigns the result to the left operand.
- AND and Assign (&=): Performs a bitwise AND operation and assigns the result to the left operand.
- OR and Assign (|=): Performs a bitwise OR operation and assigns the result to the left operand.
- XOR and Assign (^=): Performs a bitwise XOR operation and assigns the result to the left operand.
- Left Shift and Assign (<<=): Shifts bits to the left and assigns the result to the left operand.
- Right Shift and Assign (>>=): Shifts bits to the right and assigns the result to the left operand.
Operator | Description | Syntax | Example | Result |
---|---|---|---|---|
= |
Assignment | operand1 = operand2 |
a = 5 |
a is 5 |
+= |
Add and Assign | operand1 += operand2 |
a += 3 |
a is 8 |
-= |
Subtract and Assign | operand1 -= operand2 |
a -= 2 |
a is 3 |
*= |
Multiply and Assign | operand1 *= operand2 |
a *= 2 |
a is 10 |
/= |
Divide and Assign | operand1 /= operand2 |
a /= 5 |
a is 2 |
%= |
Modulus and Assign | operand1 %= operand2 |
a %= 3 |
a is 2 |
&= |
AND and Assign | operand1 &= operand2 |
a &= 3 |
a is 1 |
|= |
OR and Assign | operand1 |= operand2 |
a |= 3 |
a is 7 |
^= |
XOR and Assign | operand1 ^= operand2 |
a ^= 3 |
a is 6 |
<<= |
Left Shift and Assign | operand1 <<= operand2 |
a <<= 1 |
a is 10 |
Examples:
1. Assignment (=):
a = 5
print(a) # Output: 5
2. Add and Assign (+=):
a = 5
a += 3
print(a) # Output: 8
3. Subtract and Assign (-=):
a = 5
a -= 2
print(a) # Output: 3
4. Multiply and Assign (*=):
a = 5
a *= 2
print(a) # Output: 10
5. Divide and Assign (/=):
a = 10
a /= 5
print(a) # Output: 2.0
6. Modulus and Assign (%=):
a = 5
a %= 3
print(a) # Output: 2
7. AND and Assign (&=):
a = 5 # Binary: 0101
a &= 3 # Binary: 0011
print(a) # Output: 1 (Binary: 0001)
8. OR and Assign (|=):
a = 5 # Binary: 0101
a |= 3 # Binary: 0011
print(a) # Output: 7 (Binary: 0111)
9. XOR and Assign (^=):
a = 5 # Binary: 0101
a ^= 3 # Binary: 0011
print(a) # Output: 6 (Binary: 0110)
10. Left Shift and Assign (<<=):
a = 5 # Binary: 0101
a <<= 1
print(a) # Output: 10 (Binary: 1010)
11. Right Shift and Assign (>>=):
a = 5 # Binary: 0101
a >>= 1
print(a) # Output: 2 (Binary: 0010)
6. Other Operators:
There are many other operators:
- sizeof Operator: The sizeof operator is used to determine the size of a data type or an object in bytes. It's commonly used in languages like C and C++.
printf("Size of int: %ld\n", sizeof(int)); // Output: Size of int: 4 (may vary depending on the system)
- Comma Operator (,): The comma operator (,) allows two expressions to be evaluated in a single statement, with the left expression being evaluated first, followed by the right expression. It returns the value of the right expression.
int a, b;
a = (b = 3, b + 2);
printf("%d", a); // Output: 5
- Conditional (Ternary) Operator (? :) The conditional operator (? :) is a shorthand for an if-else statement, enabling concise conditional expressions.
int a = 10, b = 20;
int max = (a > b) ? a : b;
printf("%d", max); // Output: 20
- Dot (.) and Arrow (->) Operators: These operators are used to access members of structures and unions in C and C++. The dot (.) operator is used for direct member access, while the arrow (->) operator is used for accessing members through a pointer.
struct Point {
int x;
int y;
};
struct Point p1;
struct Point* p2;
p1.x = 10;
p1.y = 20;
p2 = &p1;
printf("%d %d\n", p1.x, p1.y); // Output: 10 20
printf("%d %d\n", p2->x, p2->y); // Output: 10 20
- Cast Operator: The cast operator is used to convert a variable from one data type to another.
int a = 5;
float b = (float)a;
printf("%f", b); // Output: 5.000000
- Address-of (&) and Dereference (*) Operators: The address-of operator (&) returns the memory address of a variable, while the dereference operator (*) is used to access the value stored at a particular memory address.
int a = 5;
int* ptr = &a;
printf("%p\n", ptr); // Output: Address of a
printf("%d\n", *ptr); // Output: 5
For more topics click here.
Happy Coding!
7 Reactions
0 Bookmarks