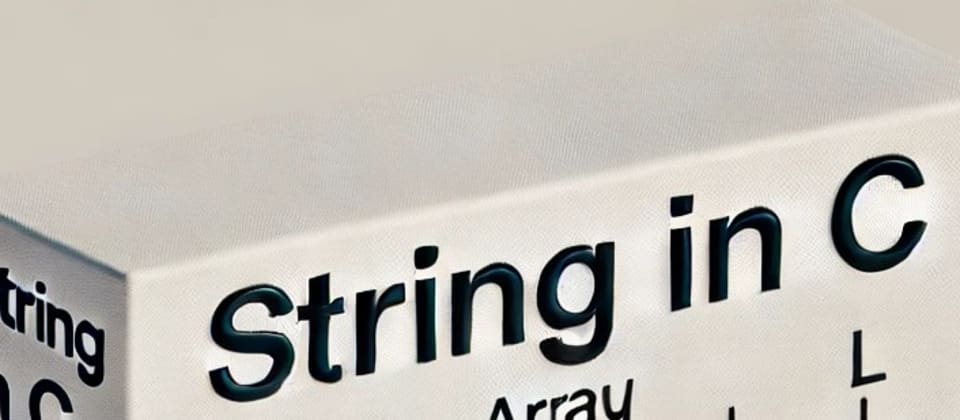
String in C
Hey Coders! Today, we are going to dive deeper into the concept of Strings in C. We'll explore the various aspects of strings. These include:
- String Initialization: for creating string variables
- String Functions: like strlen, strcpy, and strcat for handling and manipulating strings
- String Comparisons: for comparing string values using functions like strcmp.
- String I/O for reading and writing strings using functions like gets and puts.
- String Pointers: for understanding how pointers are used with strings
List of content:
- Defintion.
- Need of Strings.
- Declaration and Initialization.
- String functions.
Let's start.....
Definition:
In C, a string is essentially an array of characters terminated by a null character (\0). This null character signals the end of the string, allowing functions to determine the length and content of the string. Unlike some other programming languages, C does not have a dedicated string data type. Instead, strings in C are typically represented as arrays of characters or pointers to characters.
Need of String:
Need of strings in C Strings play a crucial role in programming, and in C, they are fundamental for several reasons:
- Data Representation: Strings are used to represent text data such as names, messages, and any sequence of characters.
- User Input:: They allow programs to accept and process user input, which is often in the form of text.
- Output Formatting: Strings are essential for displaying formatted output, including messages, prompts, and data reports.
- File Handling: Strings are used for reading from and writing to files, making it easier to process text-based data files.
- Communication: In networking and communication protocols, strings are often used to send and receive messages between systems.
- Code Management: They help in managing and manipulating text data efficiently within the program through various string functions.
Declaration of String:
In C, a string can be declared using an array of characters or a pointer to a character. Here are two common ways to declare a string:
1. Character Array:
char str[20];
This declares an array of 20 characters that can hold a string with a maximum length of 19 characters (including the null terminator).
2. Pointer to a Character:
char *str;
This declares a pointer to a character that can point to a string.
Initialization of Strings:
Once declared, you can initialize strings in different ways:
1. Using Character Array:
char str1[] = "Hello";
This initializes the array str1 with the string "Hello". The array size is automatically determined based on the length of the string (including the null terminator).
2. Specifying Array Size:
char str2[20] = "Hello";
This initializes the array str2
with the string "Hello" and reserves space for up to 20 characters.
3. Using Pointer to a Character:
char *str3 = "Hello";
This initializes the pointer str3
to point to the string literal "Hello". Note that the string literal is stored in read-only memory, so you should not modify it.
4. Manual Initialization:
char str4[6] = {'H', 'e', 'l', 'l', 'o', '\0'};
This explicitly initializes each character in the array, including the null terminator.
Example:
Here's a simple example that combines declaration and initialization:
#include <stdio.h>
int main() {
char str1[] = "Hello, World!";
char str2[20] = "Goodbye!";
char *str3 = "Welcome!";
printf("str1: %s\n", str1);
printf("str2: %s\n", str2);
printf("str3: %s\n", str3);
return 0;
}
In this example:
str1
is a character array initialized with "Hello, World!".
str2
is a character array initialized with "Goodbye!" and has extra space for more characters.
str3
is a pointer to a character initialized with "Welcome!".
Reading and Printing the string:
Reading Strings:
1. Using scanf
:
#include <stdio.h>
int main() {
char str[100];
printf("Enter a string: ");
scanf("%s", str); // Reads input until a whitespace is encountered
printf("You entered: %s\n", str);
return 0;
}
Note: scanf
reads input until it encounters a whitespace, so it won't read strings with spaces correctly.
2. Using gets
:
#include <stdio.h>
int main() {
char str[100];
printf("Enter a string: ");
gets(str); // Reads the entire line including spaces (unsafe, use fgets instead)
printf("You entered: %s\n", str);
return 0;
}
Note: gets
is unsafe and can cause buffer overflow. It's better to use fgets
.
3. Using fgets:
#include <stdio.h>
int main() {
char str[100];
printf("Enter a string: ");
fgets(str, sizeof(str), stdin); // Reads the entire line including spaces
printf("You entered: %s\n", str);
return 0;
}
Note: fgets
is safer as it prevents buffer overflow by specifying the maximum length.
4. Using Scanset:
scanf("%[^set]s", str);
Here, the [^set]
defines a scanset. The ^
indicates that scanf should read characters until one of the specified characters (in set) is encountered. Without the ^
, scanf would read only the specified characters.
Examples of Scanset:
1. Read Until a Space:
#include <stdio.h>
int main() {
char str[100];
printf("Enter a string: ");
scanf("%[^ ]", str); // Reads until a space is encountered
printf("You entered: %s\n", str);
return 0;
}
In this example, scanf
reads input until a space is encountered.
2. Read Until a Comma:
#include <stdio.h>
int main() {
char str[100];
printf("Enter a string: ");
scanf("%[^,]", str); // Reads until a comma is encountered
printf("You entered: %s\n", str);
return 0;
}
In this example, scanf
reads input until a comma is encountered.
3. Read Until a Newline:
#include <stdio.h>
int main() {
char str[100];
printf("Enter a string: ");
scanf("%[^\n]", str); // Reads until a newline is encountered
printf("You entered: %s\n", str);
return 0;
}
In this example, scanf
reads input until a newline (Enter key) is encountered.
Printing Strings:
1. Using printf
:
#include <stdio.h>
int main() {
char str[] = "Hello, World!";
printf("%s\n", str); // Prints the string
return 0;
}
2. Using puts
:
#include <stdio.h>
int main() {
char str[] = "Hello, World!";
puts(str); // Prints the string with a newline at the end
return 0;
}
String Functions:
1. strlen
: Calculates the length of a string (excluding the null terminator).
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, World!";
int length = strlen(str);
printf("Length of the string: %d\n", length);
return 0;
}
Output:
Length of the string: 13
2. strcpy
: Copies one string to another.
#include <stdio.h>
#include <string.h>
int main() {
char source[] = "Hello, World!";
char destination[20];
strcpy(destination, source);
printf("Copied string: %s\n", destination);
return 0;
}
Output:
Copied string: Hello, World!
3. strcat
: Concatenates (appends) one string to another.
#include <stdio.h>
#include <string.h>
int main() {
char str1[20] = "Hello, ";
char str2[] = "World!";
strcat(str1, str2);
printf("Concatenated string: %s\n", str1);
return 0;
}
Output:
Concatenated string: Hello, World!
4. strcmp
: Compares two strings lexicographically.
#include <stdio.h>
#include <string.h>
int main() {
char str1[] = "Hello";
char str2[] = "World";
int result = strcmp(str1, str2);
if (result == 0) {
printf("Strings are equal\n");
} else if (result < 0) {
printf("str1 is less than str2\n");
} else {
printf("str1 is greater than str2\n");
}
return 0;
}
Output:
str1 is less than str2
5. strncpy
: Copies a specified number of characters from one string to another.
#include <stdio.h>
#include <string.h>
int main() {
char source[] = "Hello, World!";
char destination[20];
strncpy(destination, source, 5);
destination[5] = '\0'; // Null-terminate the destination string
printf("Copied string: %s\n", destination);
return 0;
}
Output:
Copied string: Hello
6. strncat
: Concatenates a specified number of characters from one string to another.
#include <stdio.h>
#include <string.h>
int main() {
char str1[20] = "Hello, ";
char str2[] = "World!";
strncat(str1, str2, 3);
printf("Concatenated string: %s\n", str1);
return 0;
}
Output:
Concatenated string: Hello, Wor
7.strchr
: Finds the first occurrence of a character in a string.
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, World!";
char *ptr = strchr(str, 'W');
if (ptr) {
printf("Found 'W' at position: %ld\n", ptr - str);
} else {
printf("'W' not found\n");
}
return 0;
}
Output:
Found 'W' at position: 7
8. strstr
: Finds the first occurrence of a substring in a string.
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, World!";
char *ptr = strstr(str, "World");
if (ptr) {
printf("Found 'World' at position: %ld\n", ptr - str);
} else {
printf("'World' not found\n");
}
return 0;
}
Output:
Found 'World' at position: 7
For more topics click here.
Happy Coding!
4 Reactions
1 Bookmarks