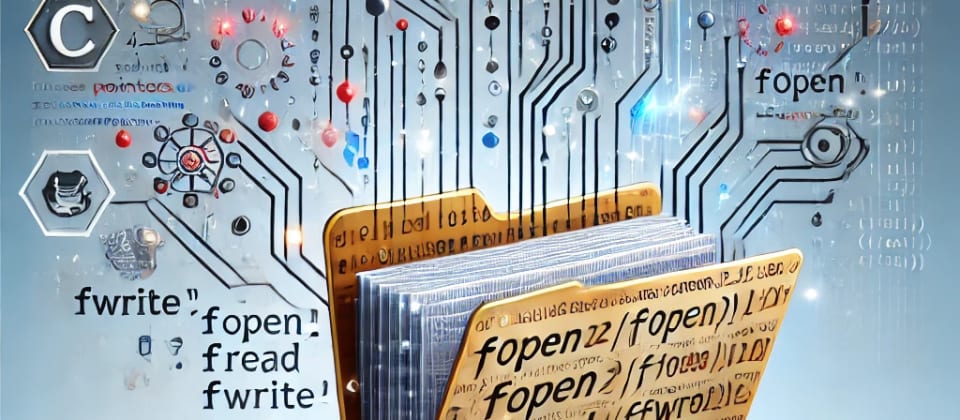
File Handling in C
Hey Coders! Today, we are going to dive deeper into the concept of file handling in C. We'll explore various aspects of file handling, including its definition and purpose, how to open and close files, reading from and writing to files using different modes, performing operations on file contents, understanding file pointers for advanced usage, and using various file handling functions provided by the standard library to efficiently manage data stored in files.
List of content:
- Definition.
- Need of File Handling.
- Types of Operations.
- Modes in File handling.
- Examples.
Let's start.....
Definition:
In C programming, file handling refers to the process of creating, opening, reading, writing, closing, and manipulating files using functions provided by the C standard library. It allows programs to interact with files on the disk, enabling data storage, retrieval, and management. File handling operations involve file pointers, modes (such as reading, writing, and appending), and various file handling functions (like fopen
, fclose
, fread
, fwrite
, etc.) to perform these tasks efficiently.
Need of File handling:
File handling in C is essential for various reasons:
- Data Storage: Programs often need to store data persistently. File handling allows saving data to disk, so it remains available even after the program exits.
- Data Retrieval: Programs can read data from files, making it possible to process information previously saved or provided by other applications.
- Data Management: File handling enables efficient management of large volumes of data, which is impractical to handle solely in memory.
- Interoperability: Programs can exchange data with other applications or systems via files, enhancing interoperability and data integration.
- User Interaction: File handling supports user-generated data input and output, allowing users to save their work, preferences, or configurations.
Types of Operations:
1. Opening a file:
In C, the fopen
function is used to open a file. This function requires two arguments:
- The name of the file.
- The mode in which the file is to be opened.
Example:
#include <stdio.h>
#include <stdlib.h>
void main() {
// Opening a file in write mode
FILE *file = fopen("example.txt", "w");
if (file == NULL) {
printf("Error opening file");
return;
}
// File opened successfully
printf("File opened successfully\n");
// Closing the file
fclose(file);
}
Output:
File opened successfully
Modes in File handling:
Mode | Description |
---|---|
"r" |
Opens a file for reading. The file must exist. |
"w" |
Opens a file for writing. If the file exists, its contents are overwritten. If the file does not exist, it is created. |
"a" |
Opens a file for appending. Data is added to the end of the file. If the file does not exist, it is created. |
"r+" |
Opens a file for both reading and writing. The file must exist. |
"w+" |
Opens a file for both reading and writing. If the file exists, its contents are overwritten. If the file does not exist, it is created. |
"a+" |
Opens a file for both reading and appending. Data is added to the end of the file. If the file does not exist, it is created. |
Example of how to open a file using different modes:
#include <stdio.h>
#include <stdlib.h>
void main() {
// Opening a file in read mode
FILE *file = fopen("example.txt", "r");
if (file == NULL) {
perror("Error opening file in read mode");
// Trying to open the file in write mode if it doesn't exist
file = fopen("example.txt", "w");
if (file == NULL) {
perror("Error opening file in write mode");
return;
} else {
printf("File created and opened in write mode\n");
}
} else {
printf("File opened in read mode\n");
fclose(file);
}
// Opening a file in append mode
file = fopen("example.txt", "a");
if (file == NULL) {
perror("Error opening file in append mode");
return;
} else {
printf("File opened in append mode\n");
}
// Writing some content to the file
fprintf(file, "Appending new content to the file.\n");
// Closing the file
fclose(file);
}
Output:
Error opening file in read mode: No such file or directory
File created and opened in write mode
File opened in append mode
If the file already exists, the output might be:
File opened in read mode
File opened in append mode
2. Writing to a File:
The fprintf
function is commonly used to write formatted data to a file. You can also use fwrite
for binary data.
#include <stdio.h>
#include <stdlib.h>
void main() {
// Opening a file in write mode
FILE *file = fopen("example.txt", "w");
if (file == NULL) {
perror("Error opening file");
return;
}
// Writing to the file
fprintf(file, "Hello, World!\n");
fprintf(file, "This is a file handling example in C.\n");
// Closing the file
if (fclose(file) == 0) {
printf("File closed successfully\n");
} else {
perror("Error closing file");
}
}
Output:
Hello, World!
This is a file handling example in C.
Examples:
Program to Create a File, Write in it, And Close the File:
#include <stdio.h>
#include <stdlib.h>
void main() {
// Opening a file in write mode
FILE *file = fopen("example.txt", "w");
if (file == NULL) {
perror("Error opening file");
return;
}
// Writing to the file
fprintf(file, "Hello, World!\n");
fprintf(file, "This is a file handling example in C.\n");
// Closing the file
if (fclose(file) == 0) {
printf("File closed successfully\n");
} else {
perror("Error closing file");
}
}
Output:
Hello, World!
This is a file handling example in C.
Program to Open a File, Read from it, And Close the File:
#include <stdio.h>
#include <stdlib.h>
void main() {
// Opening the file in read mode
FILE *file = fopen("example.txt", "r");
if (file == NULL) {
perror("Error opening file");
return;
}
// Reading from the file
char buffer[100];
while (fgets(buffer, sizeof(buffer), file) != NULL) {
printf("%s", buffer);
}
// Closing the file
if (fclose(file) == 0) {
printf("File closed successfully\n");
} else {
perror("Error closing file");
}
}
Output:
Hello, World!
This is a file handling example in C.
File closed successfully
4 Reactions
0 Bookmarks