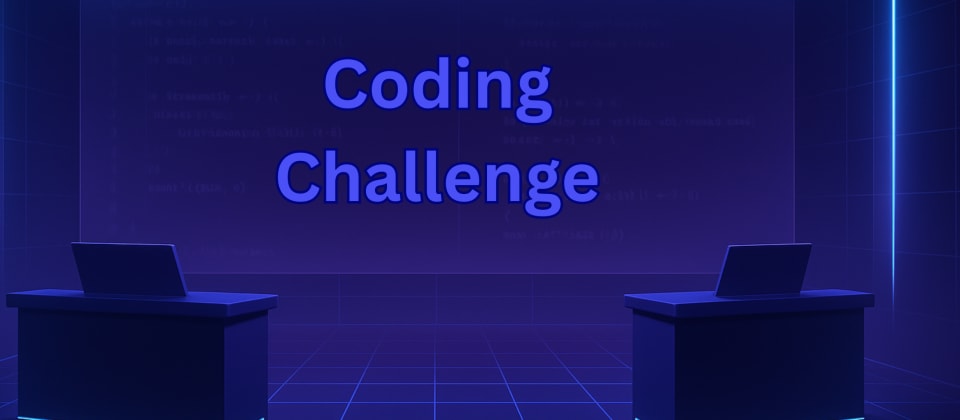
Coding Challenge - 1:
Problem Statement: Staircase
Description:
You need to write a program that prints a staircase of size n using # symbols and spaces. The staircase is right-aligned, meaning the last line is not preceded by any spaces. Both the base and height of the staircase are equal to n.
Input Format:
- A single integer n, denoting the size of the staircase.
Constraints:
- 0<𝑛≤100
Output Format:
- Print a staircase of size n using # symbols and spaces.
Input:
Function Signature:
6
Output:
Function Signature:
#
##
###
####
#####
######
Explanation: The staircase is right-aligned, composed of # symbols and spaces, and has a height and width of n = 6.
.
.
.
.
.
.
.
.
.
Try it Yourself first:
.
.
.
.
.
.
.
.
Example Solution in C++:
Function Signature:
#include <iostream>
using namespace std;
void staircase(int n) {
for(int row=1;row<=n;row++){
for(int col=1;col<=n;col++){
if((row+col)>n)
cout<<"#";
else
cout<<" ";
}
cout<<endl;
}
}
int main() {
int n;
cout << "Enter the size of the staircase: "<<endl;
cin >> n;
if (n > 0 && n <= 100) {
staircase(n);
} else {
cout << "Invalid input. Please enter a number between 1 and 100." << endl;
}
return 0;
}
Explanation:
The function staircase takes an integer n as a parameter.
It uses nested loops to print the staircase:
-
The outer loop runs from 1 to n, creating each level of the staircase.
-
The first inner loop prints the required number of spaces.
-
The second inner loop prints the # symbols.
In the main function, the user is prompted to enter the size of the staircase.
- The input is validated to ensure it is within the specified range.
This code will create a staircase of the given size, right-aligned, with # symbols and spaces.
10 Reactions
3 Bookmarks