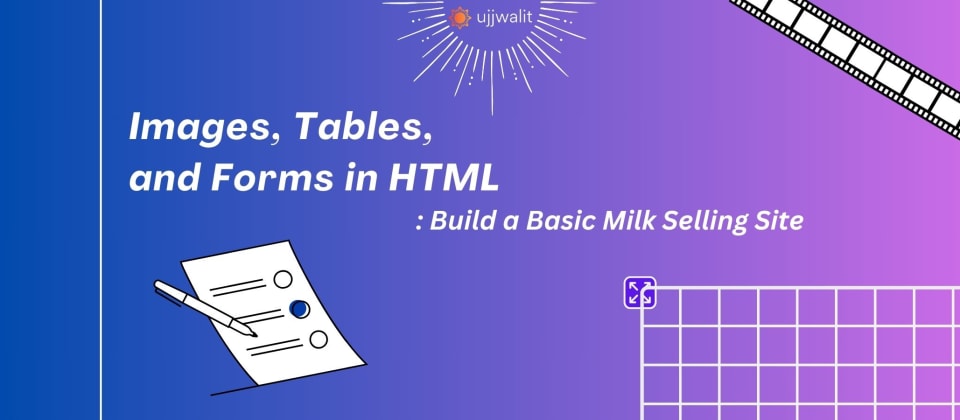
Images, Tables, and Forms in HTML: Build a Basic Milk Selling Site
Hey Devs!
Do you see the like and bookmark buttons at the bottom of the screen? You must have seen similar options on other services. It is a way to get feedback from the user to see how a particular website or service performs. To demonstrate some data or show comparisons, many platforms include tables and images to represent data in a visually appealing manner. Today we are going to cover both of these aspects by building a very basic milk-selling site.
We will cover:
- Images in HTML
- Tables in detail
- Introduction to forms
- Different Input types
Prerequisites for this blog:
- HTML basic typographic tags
- Lists
- Block and inline elements
- Closing and empty tags
If you are unfamiliar with these, check out my blog on my profile or follow this link here.
Setting up the project:
- Create a file called
index.html
and open it in VS Code or any other code editor of your choice. We will be using VS Code for this case. - Set up the HTML boilerplate and change the title to Order Milk.
- Copy and save this image. as
milk.jpeg
in the root folder (the folder where yourindex.html
file is present): - Now add three
div
tags to the HTML body. - In each
div
, add anh2
tag with the text MILK, Nutrition Table, and Order Here. - Launch the live server and see the page in the browser.
Now you are set up to work on the project.
Images in HTML
Let's start from the first div
.
We will add an image here and a paragraph about the image. To add an image, we can use the img
tag in HTML. The img
tag is an empty tag that requires one attribute: src
. In the src
attribute, we write the source of the image. You can use this link if you wish to use it directly from the web
Or specify the image path by typing:
src="./milk.jpeg"
The ./
specifies that the HTML file should look in the same folder. You can add ..
to go up a folder. For example, if your HTML file is in a folder called html
and your image is outside of the folder, you will use:
../milk.jpeg
.
Now review the image on the webpage. You will see that the image is not of an appropriate size—it is too big for the screen. You can use CSS to fix it, or you can simply give it the height
and width
attributes.
Specify the height as height="250"
. HTML calculates the value in pixels (that's the primary unit), so height="250"
means 250 pixels. You can similarly use the width
element to specify the width of the image.
Try giving it a width of 250. The image becomes stretched, which we don't like, so we can just leave it as height
. It is a good practice to declare the height and width of an image, as it improves the SEO of your webpage. A good SEO score helps search engines find your pages easily.
Remove the width
attribute and add an alt
attribute to the image. The alt
attribute increases the accessibility of your site. Give the alt
attribute the value of Milk_image. Now, for a moment, set the src
value to null
or ""
. You will see the text written as Milk_image on your browser.
The alt
attribute helps you write a brief alternative description of your image just in case the browser is unable to load your image from the source.
Now set the src
value back to what it was before. Recheck the image, and just now you learned how to include images in your HTML.
Figure and Figcaption Tags
Now it's time to learn two important tags: figure
and figcaption
.
The figure
tag is a semantic tag used to contain figures like images. The figcaption
tag allows you to declare captions for your images.
Add a figure
tag and move the img
tag into it. Add a figcaption
with the text Milk image.
Your first div
code must look like this:
<div>
<h2>MILK</h2>
<figure>
<img src="https://images.pexels.com/photos/248412/pexels-photo-248412.jpeg" alt="Milk_image" height="250">
<figcaption>Milk image</figcaption>
</figure>
</div>
Table in HTML
Now, in the second div
below the h2
heading, add a table
tag.
The table
tag in HTML is used to create a table.
You will not be able to see the table borders for columns and rows, but they can be defined by using CSS or the border
attribute.
Write the border
attribute with its value as 1
.
In the table, use the caption
tag to specify the caption for your table. Nest the Nutrition Table heading in the caption
tag.
A table can be divided into two parts:
- Table header (
thead
) - Table body (
tbody
)
Add a thead
and tbody
tag below the caption
tag.
A table can be divided into rows and columns. To create a row, we use the tr
tag. Add one tr
tag to the thead
and six tr
tags to the tbody
. Now each row needs to have some data elements. For those, we use th
and td
.
th
is used to specify the column heading. Create three th
tags in the tr
of the thead
and three td
tags in each of the tr
in the tbody
.
Now, fill the details from this table:
Nutrient | Amount per 1 cup (240 ml) | % Daily Value (DV)* |
---|---|---|
Calories | 149 kcal | 7% |
Protein | 7.7 g | 15% |
Total Fat | 8 g | 10% |
Saturated Fat | 4.6 g | 23% |
Cholesterol | 24 mg | 8% |
Carbohydrates | 12 g | 4% |
Add a p
tag with an em
tag and add the following text:
% Daily Value (DV) is based on a 2,000-calorie diet and may vary depending on individual needs.
Great! You are done with the table. Try experimenting by adding new rows and data to it.
Your table code will look like this:
<div>
<table border=1>
<caption><h2>Nutrition Table</h2></caption>
<thead>
<tr>
<th>Nutrient</th>
<th>Amount per 1 cup (240 ml)</th>
<th>% Daily Value (DV)*</th>
</tr>
</thead>
<tbody>
<tr>
<td>Calories</td>
<td>149 kcal</td>
<td>7%</td>
</tr>
<tr>
<td>Protein</td>
<td>7.7 g</td>
<td>15%</td>
</tr>
<tr>
<td>Total Fat</td>
<td>8 g</td>
<td>10%</td>
</tr>
<tr>
<td>Saturated Fat</td>
<td>4.6 g</td>
<td>23%</td>
</tr>
<tr>
<td>Cholesterol</td>
<td>24 mg</td>
<td>8%</td>
</tr>
<tr>
<td>Carbohydrates</td>
<td>12 g</td>
<td>4%</td>
</tr>
</tbody>
</table>
<p><em>% Daily Value (DV) is based on a 2,000-calorie diet and may vary depending on individual needs.</em></p>
</div>
Form in HTML
In HTML, a form is a structure used to collect user input and send it to a server for processing. Forms are essential for creating interactive websites, as they allow users to input data such as text, selections, and files.
The basic syntax of a form is:
<form action="url" method="post">
<!-- Form elements like input fields go here -->
</form>
Key Attributes of the <form>
Element:
-
action:
Specifies the URL where the form data will be sent for processing.
Example:action="https://example.com/submit"
-
method:
Defines the HTTP method used to send the form data.
Common values:GET
: Used to retrieve or request data from a server. It appends form data to the URL as query parameters (used for non-sensitive data).POST
: Used to send data to the server for processing or storing. It sends form data in the HTTP request body (preferred for sensitive or large data).
-
target:
Specifies where to display the response from the server.
Common values:_self
(default): Same window._blank
: New window or tab._parent
: Parent frame._top
: Full body of the window.
-
autocomplete:
Indicates whether the browser should enable autofill for the form fields.
Values:on
(default) oroff
.
And that's how you create a form element. You would also use other attributes in the future while working with JavaScript, but for now, these should be enough to create our project.
For now, set the action="#"
—this will redirect the form data to the same page. You can remove any other attributes for now; you can experiment with them later on.
It is better to structure your form to improve accessibility.
Use the fieldset
tag in the form.
Now in the fieldset
, create two more fieldset
tags. These help you create a proper section for your form by providing borders.
Now move the h2
heading into the outer fieldset
.
We will use a special tag called the legend
tag. The legend
tag is used to specify the name of the fieldset
. Create a legend
tag and put the h2
heading in it. See the changes on your browser—notice how the text is above the border.
Do the same for both the internal fieldsets
. In the first, add an h3
heading with the text Basic Info in the first and Order Details in the second.
Your code will look like this:
<form action="#">
<fieldset>
<legend><h2>Order Here</h2></legend>
<fieldset>
<legend><h3>Basic Info</h3></legend>
</fieldset>
<fieldset>
<legend><h3>Order Details</h3></legend>
</fieldset>
</fieldset>
</form>
Input Fields in Forms
Now, your form needs input fields to accept user input.
In HTML, there are various input types: text
, number
, email
, checkboxes
, and many others. For our site, we will take the customer's name, phone number, email, and age as input in the Basic Info fieldset, and delivery address in the Order Details fieldset. We will also add an input type submit in the outer container fieldset.
Here is a list of all the input types in HTML:
Input Type | Description |
---|---|
text |
Defines a single-line text input field. |
password |
Defines a password field, where characters are masked. |
email |
Defines a field for entering an email address. |
number |
Defines a field for entering a numeric value. |
tel |
Defines a field for entering a telephone number. |
url |
Defines a field for entering a URL. |
checkbox |
Defines a checkbox for selecting multiple options. |
radio |
Defines a radio button for selecting one option. |
date |
Defines a field for selecting a date. |
time |
Defines a field for selecting a time. |
datetime-local |
Defines a field for selecting both date and time. |
file |
Defines a field for uploading files. |
color |
Defines a color picker. |
range |
Defines a slider control for numeric range input. |
search |
Defines a search field. |
button |
Defines a clickable button. |
submit |
Defines a button for submitting a form. |
reset |
Defines a button for resetting the form fields. |
For today, we will be working with input types: text
, email
, tel
, number
, and radio
. We would also be using one different input element named textarea
.
Add input fields with the type text
, tel
, email
, and number
in the Basic Info part.
Add a p
tag before each of the input tags. Add the text "Full Name", "Phone Number", "Email", and "Age" to all of them respectively.
Now, in the second fieldset
, add a textarea
tag. The textarea
tag creates a bigger input field for the user to enter information. It is resizable—see that small arrow at the bottom right. You can drag it to resize it according to your liking or specify a proper area by setting the rows
and cols
attributes, both of which stand for rows and columns respectively. Give it a rows
value of 5 and a cols
value of 25.
You can also use a placeholder
attribute to display some placeholder text. Add a placeholder
attribute to the textarea
and give it the following value: "Enter the delivery address here."
Finally, in the outer fieldset
, add an input of type submit
.
You code will look like this:
<div>
<form action="#">
<fieldset>
<legend><h2>Order Here</h2></legend>
<fieldset>
<legend><h3>Basic Info</h3></legend>
<p>Full Name</p>
<input type="text">
<p>Phone Number</p>
<input type="tel">
<p>Email</p>
<input type="email">
<p>Age</p>
<input type="number">
</fieldset>
<fieldset>
<legend><h3>Order Details</h3></legend>
<textarea placeholder="Enter the delivery address here." rows="5" cols="25"></textarea>
</fieldset>
<input type="submit">
</fieldset>
</form>
</div>
And...
You did it!
You created a basic product description webpage by using only HTML. Try practicing and experimenting more with the knowledge gained today.
But We aren't done yet!
More About Forms
When working with forms in HTML, assigning unique IDs to input elements and associating them with labels is a best practice. This ensures better accessibility, usability, and clarity in your code.
What is an ID in HTML?
An ID is a unique identifier assigned to an HTML element. It plays a crucial role in:
- Uniquely Identifying an Element:
IDs help browsers quickly locate a specific element on the page. - Linking Labels to Inputs:
IDs are used to associate<label>
tags with their corresponding<input>
fields. - Referencing Elements in JavaScript or CSS:
IDs allow you to directly style or manipulate specific elements.
Imagine trying to locate a student in a school. If many students share the same name, you’d need their roll number to uniquely identify them. Similarly, an ID in HTML uniquely identifies an element, saving the browser from searching through all elements.
Example of an ID:
<input type="text" id="username">
What is a Label in HTML?
The <label>
tag is used to describe an <input>
field, making it easier for users to understand what information is required. Labels are essential for:
- Improving Usability:
Labels give users a clear description of the input field. - Enhancing Accessibility:
Labels make forms accessible to users who rely on screen readers.
The for
attribute in a <label>
tag links the label to the corresponding <input>
using the input's ID. Clicking on the label will automatically focus the associated input field.
Example of a Label:
<label for="username">Username:</label>
<input type="text" id="username">
Why Use IDs and Labels Together?
-
Accessibility:
Labels ensure screen readers announce the input's purpose, improving the experience for visually impaired users. -
Click Behavior:
Clicking on the label automatically focuses the corresponding input field. -
Clarity and Structure:
IDs make it easier to organize and reference form elements, improving readability and maintainability.
Implicit vs. Explicit Labeling
There are two ways to associate a label with an input field:
-
Explicit Labeling (Using the
for
Attribute):
The label explicitly refers to the input using thefor
attribute, which matches the input'sid
.Example:
<label for="username">Username:</label> <input type="text" id="username">
-
Implicit Labeling (Wrapping the Input):
The label implicitly associates with the input by wrapping it.Example:
<label> Username: <input type="text" name="username"> </label>
Key Differences:
- Both approaches are valid.
- Explicit labeling is more flexible and is generally recommended, especially for larger or more complex forms.
Benefits of Using IDs and Labels
- Improved Accessibility: Better support for assistive technologies like screen readers.
- Better Usability: Users can interact with the form more intuitively.
- Clearer Code Structure: IDs and labels enhance code organization, making it easier to maintain and debug.
Try incorporating id's and labels to the page you made today by yourself!.
We will learn more about other media tags, like video and audio, in further blogs.
Until then, keep practicing and happy learning!
4 Reactions
0 Bookmarks