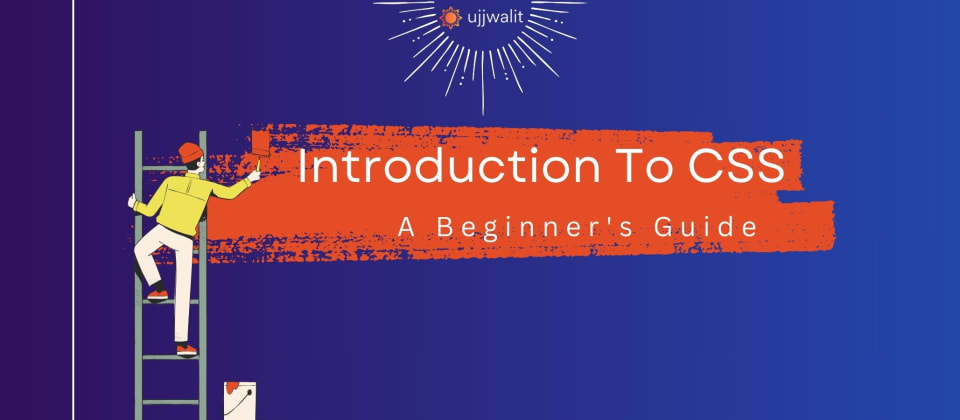
Introduction to CSS: A Beginner's Guide
Hey Devs!
Today, we are going to learn CSS (Cascading Style Sheets). We'll discuss different methods of using CSS in your HTML code and how to utilize selectors in CSS. This will be an artistic and creative beginning, so let's start our learning journey.
Prerequisites for this blog
- A basic understanding of HTML and its syntax.
If you wish to revise this, refer to this blog here.
Table of Contents
- What is CSS?
- Types of CSS
- Inline CSS
- Internal CSS
- External CSS
- Types of Selectors
What is CSS?
CSS stands for Cascading Style Sheets. It is a stylesheet language used to describe the presentation of a document written in HTML or XML. CSS defines how elements should be rendered on screens, paper, or other media. By using CSS, you can control the layout, colors, fonts, spacing, and overall visual appearance of your web pages.
Types of CSS
You can write CSS in multiple ways. You can use libraries like TailwindCSS or Bootstrap, or you can learn how to write your own CSS. We will begin by learning the traditional method.
To write your own CSS, there are three methods:
- Inline CSS
- Internal CSS
- External CSS
Inline CSS
Inline CSS is simple. You can take any HTML element and use a style
attribute with it. In the style
attribute, you can define its styles, such as color
, background-color
, and font-size
.
Here’s an example:
<h1 style="color: red; background-color: purple; font-size: 24px;">
This will render red text on a purple background with a size of 24px.
</h1>
Note: Inline CSS is not used frequently because it violates an important rule of development called the DRY principle (Do Not Repeat Yourself).
If your code contains multiple elements of the same type, you will need to write the CSS for them repeatedly, which is inefficient and discouraged. Inline CSS is best for testing or applying quick, temporary changes to a specific element.
Internal CSS
To avoid repetition, you can write internal CSS. To write internal CSS, use the <style>
tag.
Note: The
<style>
tag is a closing tag.
Here’s an example template:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>CSS Introduction | Ujjwalit</title>
<style>
/* Write your styles here */
</style>
</head>
<body>
<!-- Your HTML code goes here -->
</body>
</html>
Within the <style>
tag, you can use selectors to target either a particular element or multiple elements of the same type.
Example:
/* Targets all h1 tags */
h1 {
color: red;
font-size: 24px;
}
/* Gives the body tag a purple background */
body {
background-color: purple;
}
Why use Internal CSS?
Internal CSS is better than inline CSS because it allows you to apply style changes to multiple elements at once. However, as your CSS grows longer, it can increase the size of your HTML file. Also, while internal CSS can follow DRY for a single document, it is limited to that document only.
If you have multiple HTML pages in a project, you would need to copy and paste the same internal CSS <style>
block across all pages, which violates DRY at a project level.
To follow the Separation of Concerns principle (dividing code into separate files based on functionality) and the DRY principle, it’s better to use External CSS.
External CSS
External CSS involves linking a separate CSS file to your HTML document. Use the <link>
element in the <head>
section of your HTML.
Example:
<link rel="stylesheet" href="css_styles.css">
- The
rel
attribute specifies the type of file being linked. - The
href
attribute contains the relative path to your CSS file.
To use external CSS, create a file with a .css
extension and link it to your HTML document. External CSS follows the same syntax as internal CSS but allows for better organization and reusability.
Comparison Between Inline, Internal, and External CSS
Criteria | Inline CSS | Internal CSS | External CSS |
---|---|---|---|
Definition | Styles are applied directly within the style attribute of an HTML element. |
Styles are written within a <style> tag in the <head> section of the HTML document. |
Styles are written in a separate .css file and linked to the HTML document using a <link> tag. |
Syntax | <h1 style="color: red;">Text</h1> |
<style> h1 { color: red; } </style> |
<link rel="stylesheet" href="styles.css"> |
Scope | Applied to a single element. | Applied to multiple elements in the document. | Applied to multiple elements across multiple documents. |
Ease of Maintenance | Difficult to maintain as styles are tied to individual elements. | Easier to maintain within a single document. | Best for maintenance; changes in one file apply to all linked documents. |
Code Reusability | No reusability; styles must be repeated for each element. | Moderate reusability within the same document. | High reusability across multiple documents. |
Performance | Fast for small, localized changes but can bloat the HTML file if overused. | Slightly slower than inline CSS for rendering but still efficient. | Most efficient as styles are loaded once and cached by browsers. |
Use Case | Quick fixes, testing, or overriding other styles temporarily. | Styling a single page or section of a document. | Styling a website with consistent, centralized design. |
Follows DRY Principle | ❌ (Does not follow) | ✅ (Follows moderately) | ✅ (Best for DRY principle) |
Types of Selectors in CSS
CSS provides a variety of selectors to target HTML elements for styling. Let's explore them in detail:
1. Type Selector / Element Selector
The type selector targets all elements of a specific type. This is useful when you want to apply the same style to every occurrence of a particular element in your document.
Example:
h1 {
color: red;
}
h3 {
color: green;
}
This CSS will:
- Change the text color of all
<h1>
tags to red. - Change the text color of all
<h3>
tags to green.
2. Descendant Selector
The descendant selector targets all elements that are nested (descendants) within a specified parent element, regardless of how deep they are nested.
Syntax:
parent-element descendant-element {
/* Styles */
}
Example:
<div>
<h1>This is an h1 inside a div.</h1>
</div>
<section>
<h1>This is an h1 inside a section.</h1>
</section>
CSS:
div h1 {
color: red;
}
section h1 {
color: green;
}
- The
<h1>
inside the<div>
will have red text. - The
<h1>
inside the<section>
will have green text.
3. Universal Selector
The universal selector (*
) targets all elements in the document. This is particularly useful for applying global styles.
Example:
* {
color: purple;
}
This CSS will change the text color of every element on the page to purple.
You can also combine the universal selector with a descendant selector:
div * {
color: purple;
}
This will apply the color purple to all child elements of the <div>
.
4. Child Selector
The child selector (>
) targets only the direct children of a specified parent element, ignoring deeper descendants.
Example:
<div>
<h3>I am a direct child of the div.</h3>
<section>
<h3>I am a child of the section inside the div.</h3>
</section>
</div>
CSS:
div > h3 {
color: green;
}
- The
<h3>
directly inside the<div>
will have green text. - The
<h3>
inside the<section>
(nested deeper) will remain unaffected.
5. ID Selector
The ID selector targets a specific element using its unique id
attribute. IDs must be unique within a document.
Example:
<div>
<h3>This heading is red.</h3>
<h3>This heading is red too.</h3>
<h3 id="greenHeading">This heading is green because of its ID.</h3>
<h3>This heading is also red.</h3>
</div>
CSS:
/* Default style for all h3 elements */
div h3 {
color: red;
}
/* Override style for the element with the ID "greenHeading" */
#greenHeading {
color: green;
}
- All
<h3>
elements will be red except the one with theid="greenHeading"
, which will be green.
6. Class Selector
The class selector targets elements that share a common class
attribute. Unlike IDs, multiple elements can share the same class.
Example:
<div>
<h3 class="redtext">This heading is red.</h3>
<h3 class="greentext">This heading is green.</h3>
<h3 class="greentext">This heading is green too.</h3>
<h3 class="redtext">This heading is also red.</h3>
</div>
CSS:
/* Style for elements with the class "redtext" */
.redtext {
color: red;
}
/* Style for elements with the class "greentext" */
.greentext {
color: green;
}
- The first and fourth
<h3>
elements will be red. - The second and third
<h3>
elements will be green.
7. Attribute Selector
The attribute selector targets elements based on the presence or value of a specific attribute.
Example:
<form>
<input type="text" placeholder="Enter your name">
<input type="password" placeholder="Enter your password">
<input type="submit" value="Submit">
</form>
CSS:
/* Target all input elements with the type "text" */
[type="text"] {
background-color: lightgray;
}
- The
type="text"
input field will have a light gray background.
8. Adjacent Sibling Selector (+
)
The adjacent sibling selector targets an element that immediately follows a specified element.
Example:
<h1>Heading</h1>
<p>This is a paragraph that comes right after the heading.</p>
<p>This paragraph is not immediately after the heading.</p>
CSS:
h1 + p {
color: brown;
}
- Only the first
<p>
(immediately after<h1>
) will be styled with brown text.
9. General Sibling Selector (~
)
The general sibling selector targets all siblings of a specified element.
Example:
<h1>Heading</h1>
<p>This is the first sibling paragraph.</p>
<p>This is another sibling paragraph.</p>
CSS:
h1 ~ p {
color: brown;
}
- Both
<p>
elements following the<h1>
will be styled with brown text.
10. Combining Selectors
You can combine multiple selectors by separating them with commas.
Example:
h1, p + a, div > h3, .red {
color: red;
}
This CSS will:
- Make all
<h1>
elements red. - Style
<a>
elements that immediately follow a<p>
with red text. - Target
<h3>
elements that are direct children of a<div>
and make them red. - Apply red text to any element with the class
red
.
Specificity and Overriding in CSS
CSS follows a specificity hierarchy to decide which styles take precedence when multiple rules target the same element. The specificity of a selector is determined by the following:
- Inline CSS (Most specific)
- ID Selectors
- Class, Attribute, and Pseudo-class Selectors
- Element and Pseudo-element Selectors
- Universal Selector (
*
) (Least specific)
Selectors with higher specificity override those with lower specificity. If specificity is tied, the last defined rule in the CSS or HTML document will take precedence (source order).
Example: Overriding Styles Based on Specificity
HTML:
<div id="example" class="container">
<h1 style="color: blue;">Hello World</h1>
</div>
CSS:
/* General element selector */
h1 {
color: green;
}
/* Class selector */
.container h1 {
color: orange;
}
/* ID selector */
#example h1 {
color: red;
}
Result:
- The
<h1>
will be styled with blue because inline styles have the highest specificity. - If the inline style (
style="color: blue;"
) is removed, the<h1>
will inherit the style from the#example
ID selector (red), as it has higher specificity than the class and element selectors.
By understanding specificity and the strengths of each CSS type, you'll be able to write clean, maintainable, and efficient styles for your projects.
Hope you now have a solid understanding of CSS selectors and how to use them effectively!
To keep getting better, join the platform to empower your learning!
See you in the next blog, until then Keep Practicing and Happy Learning!
3 Reactions
0 Bookmarks