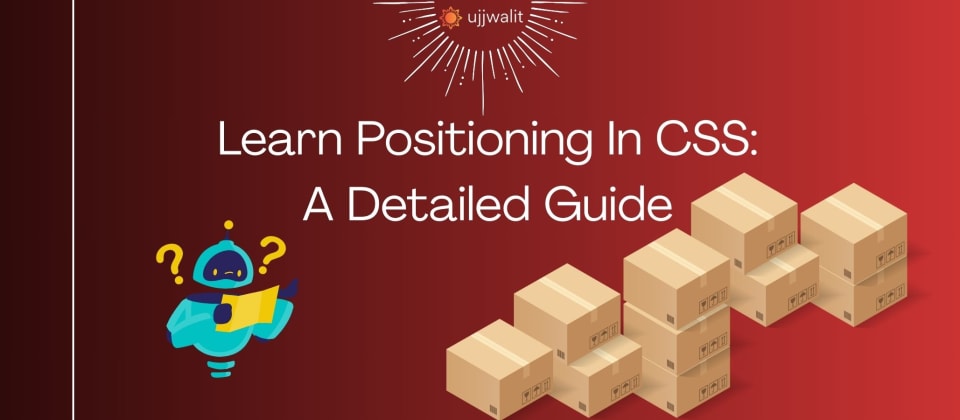
Learn Positioning In CSS: Part 1 (Static and Relative)
Hey Devs!
CSS positioning is a powerful tool that determines how elements are arranged on a webpage. It can make or break your design if not properly understood. In this in-depth guide, we’ll explore the five key CSS positioning values—static
, relative
, absolute
, fixed
, and sticky
—through a vivid parking lot analogy. We'll also dive into practical code examples, edge cases, and best practices for Static
and Relative
Positioning today.
What is CSS Positioning?
CSS positioning is a method to control the placement of elements on a webpage. It allows you to:
- Move elements relative to their default position or specific containers
- Create layered effects using z-index
- Build dynamic layouts with sticky headers, popups, and overlays
- Fine-tune visual arrangements without disturbing the flow
To make this crystal clear, let’s imagine a parking lot. Each car represents an HTML element, and the parking lot is your webpage. The way cars are parked and managed aligns perfectly with CSS positioning concepts.
Static Positioning: "Parked by Default"
Parking Lot Analogy
Imagine you arrive at a hotel parking lot, and the valet parks your car in the first available spot, following the order of arrival. Your car is neatly parked in line, and you cannot move it yourself—it stays fixed in that spot. Other cars arrive and are parked sequentially based on their order of arrival.
This scenario perfectly represents static positioning in CSS. Just like the cars in the parking lot:
- Your car’s position is determined solely by the order it arrived (the order of elements in HTML).
- You can’t reposition it using properties like
top
,right
,bottom
, orleft
. - It strictly adheres to the flow—no overlapping or adjustments allowed.
What Exactly is Static Positioning?
In CSS, position: static
is the default positioning for all elements. Even if you don’t specify a position
property, elements behave as if they are position: static
.
Characteristics of Static Positioning:
- Document Flow Adherence: Elements are positioned based on the order in the HTML document—top to bottom.
- No Offsets: Properties like
top
,right
,bottom
, andleft
do not work with static positioning. - No Overlap or Movement: The element cannot be shifted or layered over other elements using offsets.
- No Stacking Context Created: Static elements do not create a new stacking context, affecting how overlapping elements are layered.
Code Example: Basic Static Positioning
<!DOCTYPE html>
<html>
<head>
<style>
.static-box {
position: static;
width: 150px;
height: 100px;
background-color: lightblue;
border: 2px solid black;
margin-bottom: 10px;
}
.attempt-move {
position: static;
top: 20px; /* This will be IGNORED! */
left: 20px; /* This will be IGNORED! */
background-color: lightcoral;
}
</style>
</head>
<body>
<div class="static-box">I am static</div>
<div class="attempt-move">Top and Left do not work on me</div>
</body>
</html>
Explanation of the Code:
- The
.static-box
element follows the normal document flow. .attempt-move
hastop: 20px
andleft: 20px
, but those properties are ignored becauseposition: static
does not recognize them.- Both boxes are stacked vertically, respecting the flow of the document.
Result:
- The second box does not move despite the offset properties.
- Both boxes are arranged one below the other, following the order of HTML.
Common Misconceptions About Static Positioning:
-
“Why isn’t my element moving?”
This is becauseposition: static
ignores offset properties. To move elements, userelative
,absolute
,fixed
, orsticky
instead. -
“Can I layer static elements?”
No, because static elements do not create a stacking context. Useposition: relative
orabsolute
if you need overlapping. -
“Why is my element not responding to top and left?”
Thetop
,right
,bottom
, andleft
properties only work with positioned elements (relative
,absolute
,fixed
,sticky
).
Practical Use Cases for Static Positioning:
- Standard Flow Content: For text, paragraphs, headings, and other content that should appear naturally in the document flow.
- Consistent Layouts: Ensures elements align and wrap naturally, ideal for sequential content.
- Default Positioning: If you don’t need custom positioning,
position: static
keeps your layout clean and predictable.
When NOT to Use Static Positioning:
- Dynamic Layouts: For interactive elements or custom overlays, use other positioning techniques.
- Floating Headers/Footers: Use
fixed
orsticky
instead. - Precise Control Needed: If you need control over the element’s exact placement, static positioning won’t cut it.
position: static
may seem limiting, but it’s perfect for elements that should follow the natural document flow. It keeps layouts clean and predictable, reducing complexity. When your content needs to interact with the viewport or overlap other elements, you’ll need one of the more advanced positioning options.
Relative Positioning: The Controlled Car
Imagine you’re back at the hotel parking lot, but this time, your car is parked in its designated spot by the valet (the document flow). You suddenly realize you need to reposition your car slightly—maybe to avoid a puddle or just to get a better view.
️ Analogy Breakdown:
- You can shift your car around—forward, backward, left, or right—but you can’t leave your parking space.
- When you reposition your car, the original space remains occupied (as if a ghost of your car is still there).
- Other cars (elements) still behave as if your car is in its original spot, even if it visually moved.
- You can’t park on top of another car, but you might visually overlap it.
This scenario is exactly how relative positioning works in CSS.
What Exactly is Relative Positioning?
position: relative
in CSS allows you to offset an element relative to its normal position in the document flow. The element remains in the flow, but you can visually shift it around.
Characteristics of Relative Positioning:
- Stays in Document Flow: The element remains in the flow, maintaining its original space.
- Offsets Are Applied Visually: Properties
top
,right
,bottom
, andleft
move the element from its original position, but space is still reserved. - Does Not Affect Neighbors: Moving the element doesn’t cause neighboring elements to adjust—layout remains unchanged.
- Creates a Positioning Context for Children: Acts as a reference point for absolutely positioned child elements.
Code Example: Basic Relative Positioning
Let’s see a straightforward example to grasp relative positioning:
<!DOCTYPE html>
<html>
<head>
<style>
.container {
width: 300px;
height: 200px;
background-color: lightgray;
margin-bottom: 20px;
}
.relative-box {
position: relative;
top: 20px;
left: 30px;
width: 150px;
height: 100px;
background-color: coral;
}
</style>
</head>
<body>
<div class="container">
<div class="relative-box">I am relatively positioned</div>
</div>
</body>
</html>
Explanation of the Code:
.container
sets the background for context but does not control positioning..relative-box
usesposition: relative
withtop: 20px
andleft: 30px
, so it moves 20px down and 30px right from its original spot.- The element still occupies its original space, but visually shifts.
Result:
- The coral box appears 20px lower and 30px to the right from its initial position.
- The original space remains occupied, maintaining the document flow.
Visualizing the "Ghost Space" Effect
Let’s modify the example to make the reserved space clearer:
<!DOCTYPE html>
<html>
<head>
<style>
.container {
width: 300px;
height: 200px;
background-color: lightgray;
position: relative;
}
.relative-box {
position: relative;
top: 20px;
left: 30px;
width: 150px;
height: 100px;
background-color: coral;
}
.neighbor {
width: 100px;
height: 100px;
background-color: lightblue;
}
</style>
</head>
<body>
<div class="container">
<div class="relative-box">Moved 20px down, 30px right</div>
<div class="neighbor">I am a neighbor</div>
</div>
</body>
</html>
Explanation of the Code:
.relative-box
visually shifts, but its original space remains..neighbor
does not adjust its position and acts as if.relative-box
is still in its original spot.- This “ghost space” effect can sometimes cause confusion!
Result:
- The coral box is offset, but
.neighbor
is positioned as if the box never moved. - This demonstrates how relative positioning doesn’t disrupt the document flow.
Relative Positioning for Context in Absolute Positioning
position: relative
is incredibly useful when combined with position: absolute
. It acts as a reference point for child elements.
<!DOCTYPE html>
<html>
<head>
<style>
.parent {
position: relative;
width: 300px;
height: 200px;
background-color: lightgray;
margin-bottom: 20px;
}
.child {
position: absolute;
top: 20px;
left: 20px;
width: 100px;
height: 100px;
background-color: coral;
}
</style>
</head>
<body>
<div class="parent">
<div class="child">Absolutely Positioned</div>
</div>
</body>
</html>
Explanation of the Code:
.parent
is positioned relatively, setting a reference point..child
is positioned absolutely relative to.parent
.- The child element appears 20px down and 20px right from the parent’s top-left corner.
Result:
- The coral box appears inside
.parent
at the specified offset. - Without
position: relative
on.parent
, the box would be offset relative to the viewport!
Common Pitfalls with Relative Positioning
- Unexpected Overlap: Offsetting an element too much can overlap neighbors, creating a messy layout.
- Confusing the Purpose: It’s often misunderstood as a way to completely reposition elements. Remember, it only shifts visually.
- Incorrect Parent Context: Using relative positioning solely to contain absolutely positioned children is a common misuse.
When to Use Relative Positioning:
- To make subtle adjustments to an element’s position without removing it from the document flow.
- When you need a reference point for absolutely positioned child elements.
- To visually move elements while maintaining their logical flow.
position: relative
is subtle yet powerful. It allows controlled, visual adjustments without affecting the document flow and sets up positioning contexts for child elements. It’s perfect for making slight adjustments and for nesting absolute elements.
Absolutely! Let’s dive deep into fixed positioning in CSS with a refined analogy, detailed explanation, and practical code examples.
Now that we’ve covered static and relative, you’ve got a solid grasp of both of these CSS positioning! Use these tools to create engaging layouts with precision. Try practicing on your own and brainstorm their use cases.
We will cover Position Absolute, Fixed, and Static in the next blog.
Until then, keep practicing and happy learning!
5 Reactions
1 Bookmarks