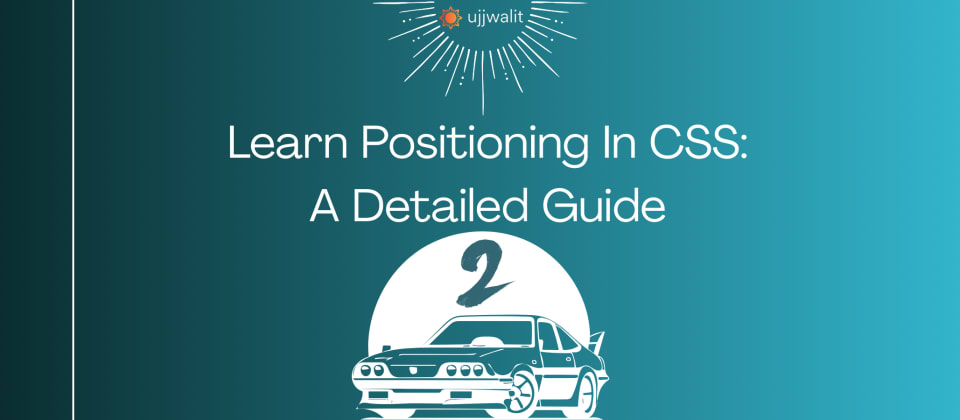
Learn Positioning In CSS: Part 2 (Absolute, Fixed & Sticky)
Hey Devs!
Today we will cover position absolute
, fixed
, and sticky
. If you wish to learn about position static
and relative
check out part 1 here.
Absolute Positioning in CSS: The Free-Roaming Car
Imagine you arrive at a parking lot where a valet (HTML document) usually parks your car according to the lot's rules. But instead of following the usual flow, your car decides it can park anywhere it wants—as long as it’s near a specific reference point.
️ Analogy Breakdown:
- If the valet (document) is not present, your car parks anywhere in the lot—ignoring all parking rules.
- However, if a VIP parking attendant (a parent element with
position: relative
,absolute
,fixed
, orsticky
) is around, your car positions itself in reference to the VIP’s location. - Your car doesn’t take up a regular parking spot; it’s "floating" in a specific location.
- Other cars act as if your car isn’t even there.
This scenario illustrates absolute positioning in CSS.
What Exactly is Absolute Positioning?
position: absolute
in CSS allows an element to be positioned relative to its closest positioned ancestor. If no ancestor has a position other than static
, it positions itself relative to the initial containing block—usually the viewport.
Characteristics of Absolute Positioning:
- Removed from Document Flow: The element is taken out of the normal flow. Other elements behave as if it doesn’t exist.
- Positions Relative to Nearest Positioned Ancestor: Looks for the nearest ancestor with
position: relative
,absolute
,fixed
, orsticky
. - Supports Offsets:
top
,right
,bottom
, andleft
properties determine the position. - Creates a Stacking Context: Controls overlapping elements using
z-index
.
Code Example: Basic Absolute Positioning
Let’s start with a simple example to understand how absolute positioning works.
<!DOCTYPE html>
<html>
<head>
<style>
.container {
position: relative;
width: 300px;
height: 300px;
background-color: lightgray;
margin-bottom: 20px;
}
.absolute-box {
position: absolute;
top: 50px;
left: 50px;
width: 150px;
height: 150px;
background-color: coral;
}
</style>
</head>
<body>
<div class="container">
<div class="absolute-box">I am absolutely positioned</div>
</div>
</body>
</html>
Explanation of the Code:
.container
is set toposition: relative
—it acts as the reference point for.absolute-box
..absolute-box
usesposition: absolute
, so it positions itself 50px from the top and 50px from the left of.container
.- The absolute box does not occupy space in the document flow, so
.container
acts as if it isn’t there.
Result:
- The orange box is placed inside the container, exactly 50px from the top and left edges.
- It would have positioned relative to the viewport if
.container
were not positioned.
Absolute Positioning Without a Positioned Ancestor
Let’s see what happens when the parent container isn’t positioned:
<!DOCTYPE html>
<html>
<head>
<style>
.container {
width: 300px;
height: 300px;
background-color: lightgray;
margin-bottom: 20px;
}
.absolute-box {
position: absolute;
top: 20px;
left: 20px;
width: 150px;
height: 150px;
background-color: coral;
}
</style>
</head>
<body>
<div class="container">
<div class="absolute-box">Positioned relative to viewport</div>
</div>
</body>
</html>
Explanation of the Code:*
.container
has noposition
set, so it defaults toposition: static
..absolute-box
can’t find a positioned ancestor, so it positions itself relative to the viewport (browser window).
Result:
- The orange box appears 20px from the top and left of the entire page, not
.container
. - This behavior can lead to unexpected placements if you forget to position the parent element.
Common Pitfalls with Absolute Positioning
- Unexpected Positioning: If the ancestor is not positioned (no
relative
,absolute
,fixed
, orsticky
), the element aligns to the viewport. - Overuse Can Break Layouts: Overusing absolute positioning can make layouts hard to maintain.
- No Space Reserved in Flow: Absolute elements don’t affect the position of surrounding elements, which can create gaps.
- Confusion with Fixed Positioning: Unlike
fixed
, absolute elements move with the page scroll unless the reference point is the viewport.
When to Use Absolute Positioning:
- Dropdown menus, popups, and tooltips.
- Floating icons or buttons in specific areas.
- Dynamic UI elements requiring precise placement.
- Overlays or modals covering content.
position: absolute
is incredibly powerful for precise control but can lead to tricky bugs if misused. Always ensure your parent container is properly positioned to avoid unexpected behavior!
Fixed Positioning in CSS: The VIP Car
Imagine a VIP car in a luxurious hotel parking lot. Unlike regular cars that need to park according to rules, the VIP car has special privileges:
- It can park anywhere it wants—in front of the entrance, on a red carpet, or even blocking the driveway.
- No matter how much the parking lot changes, the VIP car never moves. The valet can drive other cars away, expand the lot, or even rebuild the hotel, but the VIP car stays put.
- It ignores everything around it and stays fixed in place.
This describes fixed positioning in CSS—an element that sticks to a specific location on the screen, regardless of scrolling.
What Exactly is Fixed Positioning?
position: fixed
in CSS positions an element relative to the viewport. This means it remains in the same spot on the screen even when the user scrolls.
Characteristics of Fixed Positioning:
- Removed from Document Flow: The element is taken out of the normal flow, so surrounding elements behave as if it isn’t there.
- Always Relative to Viewport: It doesn’t consider parent elements—just the viewport.
- Supports Offsets:
top
,right
,bottom
, andleft
determine the position relative to the viewport. - Great for Sticky UI Elements: Ideal for headers, floating buttons, and sidebars that should stay visible.
- Scroll-Independent: Remains visible on screen, even when scrolling.
Basic Code Example: Fixed Positioning
Let’s see a straightforward example of fixed positioning in action:
<!DOCTYPE html>
<html>
<head>
<style>
.content {
height: 1500px;
padding: 20px;
}
.fixed-box {
position: fixed;
top: 20px;
right: 20px;
width: 200px;
height: 100px;
background-color: coral;
padding: 10px;
color: white;
}
</style>
</head>
<body>
<div class="fixed-box">
I'm fixed! Scroll away!
</div>
<div class="content">
<h1>Scroll down to see the effect</h1>
<p>Keep scrolling, and watch how the fixed box stays in place!</p>
</div>
</body>
</html>
Explanation of the Code:
.fixed-box
is set toposition: fixed
withtop: 20px
andright: 20px
.- It stays 20px from the top and right edges of the viewport, regardless of scrolling.
.content
provides scrollable content to test the effect.
Result:
- The coral box stays in the top-right corner even as you scroll the page.
- It’s "pinned" to the viewport, perfect for floating notifications or chat buttons!
Common Pitfalls with Fixed Positioning
- Viewport Issues on Mobile: Fixed positioning can behave unexpectedly on mobile due to virtual keyboards and dynamic viewports.
- Overlapping Content: Forgetting to manage z-index or adding padding can cause content to hide under fixed elements.
- Overuse Can Be Distracting: Too many fixed elements can clutter the screen and annoy users.
When to Use Fixed Positioning:
- Floating navigation bars or sidebars
- Persistent call-to-action buttons
- Chat widgets and floating menus
- Scroll-to-top buttons
- Notifications and alerts
position: fixed
is a fantastic way to create persistent, on-screen elements that stay visible even during scrolling. However, it needs to be used wisely to avoid obstructing content or frustrating users.
Sticky Positioning in CSS: The Hybrid Car
Imagine you’re back at the hotel parking lot, but this time, the hotel has a special VIP parking spot reserved for important guests.
️ Analogy Breakdown:
- When the parking lot is empty or partially full, your car behaves just like a regular car—parked normally in the lot.
- However, as more cars arrive (more content is scrolled), your car reaches the VIP spot and sticks to it—refusing to be pushed away by the other cars.
- If the VIP spot is cleared or not needed, your car behaves like a regular one again.
- It’s a hybrid behavior: sometimes normal, sometimes special, depending on the situation.
This describes sticky positioning in CSS—a unique blend of relative and fixed positioning.
What Exactly is Sticky Positioning?
position: sticky
in CSS is a hybrid positioning method:
- Acts like
position: relative
until a scroll threshold is crossed. - Once triggered, it behaves like
position: fixed
and sticks to a specified offset. - Great for elements like sticky headers or sidebars that stay visible while scrolling.
Characteristics of Sticky Positioning:
- Hybrid Behavior: Acts like a static/relative element by default and becomes fixed when scrolled to a certain point.
- Requires a Scroll Container: Works only within the boundaries of its closest scrollable ancestor (e.g.,
overflow: scroll
oroverflow: auto
). - Scroll-Triggered: Only sticks when scrolling pushes it to the defined offset (top, right, bottom, or left).
- Won’t Escape Scroll Container: Won’t stick if there isn’t enough scrollable content.
Code Example: Basic Sticky Positioning
Let’s create a sticky header to see it in action:
<!DOCTYPE html>
<html>
<head>
<style>
.container {
height: 1500px;
padding: 20px;
}
.sticky-header {
position: sticky;
top: 0;
background-color: coral;
padding: 15px;
font-size: 20px;
color: white;
}
</style>
</head>
<body>
<div class="sticky-header">
I'm a Sticky Header!
</div>
<div class="container">
<h1>Scroll down to see the effect</h1>
<p>Keep scrolling, and watch how the header stays in place once it reaches the top.</p>
</div>
</body>
</html>
Explanation of the Code:
.sticky-header
hasposition: sticky
andtop: 0
, so it sticks to the top of the viewport when scrolled to.- Initially, it behaves like a regular block element in the document flow.
- Once the top edge of
.sticky-header
touches the viewport’s top, it becomes fixed. - Scroll up, and it returns to normal positioning.
Result:
- The header scrolls away initially but sticks to the top once you scroll past it.
- Perfect for persistent navigation or section headers!
Sticky Positioning with a Scroll Container
Sticky positioning only works within a scrollable ancestor. Here’s an example demonstrating that behavior:
<!DOCTYPE html>
<html>
<head>
<style>
.outer-container {
width: 300px;
height: 400px;
overflow-y: scroll;
border: 2px solid black;
padding: 10px;
}
.inner-container {
height: 800px;
background-color: lightgray;
padding: 10px;
}
.sticky-box {
position: sticky;
top: 10px;
background-color: coral;
padding: 10px;
font-size: 18px;
}
</style>
</head>
<body>
<div class="outer-container">
<div class="inner-container">
<div class="sticky-box">I'm Sticky Inside My Scroll Container</div>
<p>Keep scrolling inside the box to see the sticky effect.</p>
<p>Notice how the element sticks when scrolled to the top of the container, not the viewport!</p>
</div>
</div>
</body>
</html>
Explanation of the Code:
.outer-container
is scrollable due tooverflow-y: scroll
..sticky-box
becomes sticky relative to.outer-container
when scrolled totop: 10px
.- The sticky behavior only works within the scrollable container—not the viewport.
Result:
- Scroll inside the container, and the sticky box stays in place.
- It won’t stick if the outer container is too short or has no scrollable content.
Common Pitfalls with Sticky Positioning
- Not Enough Scrollable Space: If the parent container isn’t tall enough to scroll, sticky won’t activate.
- Overflow Restrictions: Sticky positioning won’t work if the parent has
overflow: hidden
. - Browser Compatibility: Some older browsers may not fully support sticky positioning.
- Incorrect Parent Context: The sticky element only sticks within the scrollable ancestor.
When to Use Sticky Positioning:
- Persistent headers or footers that stay visible while scrolling
- Table headers that remain visible when scrolling long tables
- Sidebars or floating menus within scrollable content
- Content sections that need emphasis when visible
position: sticky
is a powerful, flexible tool for creating persistent, on-screen elements that adapt based on scroll position. It combines the best of relative and fixed positioning, offering context-aware behavior.
Now that we’ve covered static, relative, absolute, fixed, and sticky positioning, you’ve got a solid grasp of CSS positioning! Use these tools to create dynamic, engaging layouts with precision.
Sign Up Today to Keep Learning Awesome Content.
Join our WhatsApp channel by following the link here.
See you in the next blog. Until then, keep practicing and happy learning!
5 Reactions
0 Bookmarks