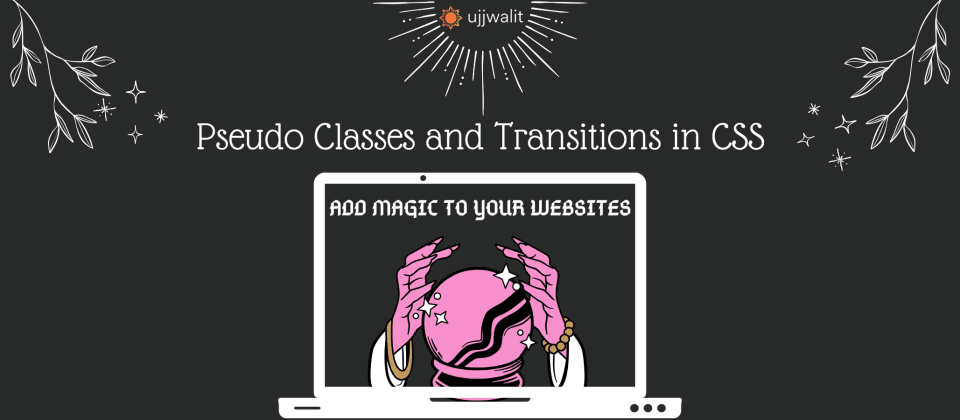
Pseudo Classes and CSS Transitions
Hey Devs
Ever wanted your website to respond to user interactions — like hover effects, form focus states, or visual feedback — without writing any JavaScript? That’s exactly what CSS pseudo-classes and transitions are built for.
Together, they allow you to create polished, interactive experiences that are clean, efficient, and easy to maintain — all with just CSS.
In this guide, we'll cover:
- What pseudo-classes are
- How transitions work
- Common timing functions
- Real-world use cases
- Tips for combining both effectively
Let’s start with pseudo-classes.
What Are CSS Pseudo-Classes?
CSS pseudo-classes let you style elements based on their state or position, without adding extra classes or modifying the HTML. You can use them to target elements that are hovered, focused, selected, invalid, or in a specific order.
They are written using a single colon (:
) before the pseudo-class name:
selector:pseudo-class {
property: value;
}
Common Pseudo-Classes You’ll Use
1. :hover
Applies when a user hovers their pointer over the element.
a:hover {
color: darkblue;
text-decoration: underline;
}
Used widely for interactive elements like buttons, links, and icons.
2. :focus
Applies when an element is focused — for example, when a user clicks into a text input.
input:focus {
border-color: steelblue;
background-color: #f0f8ff;
}
Improves usability, especially for form elements and accessibility.
3. :active
Applies while the element is in an active state (e.g., a button being clicked).
button:active {
background-color: #444;
transform: scale(0.98);
}
Useful for providing click feedback on buttons or links.
4. :nth-child()
Selects elements based on their order within a parent.
li:nth-child(odd) {
background-color: #f7f7f7;
}
This is ideal for creating alternating styles, such as striped lists or tables.
5. :checked
Targets checkboxes, radio buttons, or option elements that are selected.
input:checked + label {
font-weight: bold;
color: green;
}
Useful for styling selected form controls and toggles.
6. :not()
Excludes certain elements from selection.
button:not(.primary) {
background-color: #ccc;
}
Helpful for applying broad styles while skipping exceptions.
What Are CSS Transitions?
CSS transitions allow you to smoothly animate changes in property values over time. Without transitions, style changes happen instantly. Transitions let you control the speed and pacing of those changes, enhancing the user experience.
Syntax:
selector {
transition: property duration timing-function delay;
}
Example:
.button {
background-color: #666;
color: white;
transition: background-color 0.3s ease;
}
.button:hover {
background-color: #333;
}
Properties That Work Well with Transitions
Not all CSS properties are animatable, but many are. Common examples include:
color
background-color
opacity
transform
height
,width
border
,border-radius
margin
,padding
Understanding Transition Timing Functions
The timing function determines how the animation progresses over time — whether it starts slow and speeds up, or moves at a consistent pace.
1. ease
- Starts slow, speeds up, then slows down again.
- Good default for most UI transitions.
transition: all 0.3s ease;
2. linear
- Moves at a constant speed from start to end.
- Common for loaders and progress bars.
transition: width 1s linear;
3. ease-in
- Starts slowly and speeds up at the end.
- Works well for fade-ins or entrance animations.
transition: opacity 0.4s ease-in;
4. ease-out
- Starts quickly and slows down at the end.
- Suitable for dismissing or fading out elements.
transition: opacity 0.4s ease-out;
5. ease-in-out
- Starts slow, speeds up in the middle, and ends slow.
- Useful for interactive hover effects and modal animations.
transition: transform 0.3s ease-in-out;
6. cubic-bezier(n,n,n,n)
- Custom timing curve.
- Gives full control over animation speed and feel.
transition: all 0.5s cubic-bezier(0.25, 1, 0.5, 1);
Use tools like cubic-bezier.com to visually create and preview custom curves.
Combine Pseudo-Classes with Transitions
Combining pseudo-classes with transitions lets you create responsive, interactive styles that animate smoothly.
Example: Interactive Button
.button {
background-color: #555;
color: white;
padding: 10px 20px;
border: none;
transition: background-color 0.3s, transform 0.2s ease-in-out;
}
.button:hover {
background-color: #333;
transform: scale(1.05);
}
This button changes color and scale on hover, and the transitions control how that change feels.
Real-World Use Cases
1. Tooltip on Hover
.tooltip::after {
content: "More Info";
position: absolute;
top: -25px;
background: #333;
color: white;
padding: 4px 8px;
border-radius: 4px;
opacity: 0;
transition: opacity 0.3s ease-in;
}
.tooltip:hover::after {
opacity: 1;
}
Displays a tooltip smoothly when the user hovers.
2. Highlighting Invalid Form Inputs
input:invalid {
border-color: red;
background-color: #ffe6e6;
transition: border-color 0.3s ease-out, background-color 0.3s ease-out;
}
Provides immediate, user-friendly feedback on invalid inputs.
3. Expanding Section with Checkbox
.toggle:checked ~ .content {
max-height: 200px;
opacity: 1;
}
.content {
max-height: 0;
opacity: 0;
overflow: hidden;
transition: all 0.4s ease;
}
Creates an interactive section that expands or collapses smoothly using only CSS.
4. Progress Bar with Linear Speed
.progress {
width: 0%;
transition: width 2s linear;
}
.progress.active {
width: 100%;
}
Used to animate a progress bar consistently over a set time.
Best Practices
- Always define the
transition
on the base state, not only in the hover or active state. - Use appropriate timing functions for the interaction. Subtle and predictable transitions often feel better.
- Limit the number of properties you animate. Avoid animating layout-affecting properties (like
top
,left
,height
) on large elements for performance reasons. - Combine with pseudo-elements (
::before
,::after
) for decorative or instructional elements that don’t belong in the DOM. - Test transitions on different screen sizes and devices to ensure accessibility and responsiveness.
Conclusion
CSS pseudo-classes and transitions offer a simple, powerful way to build interactive, responsive interfaces — without needing extra JavaScript or HTML elements. They allow your design to react to user behavior while keeping your markup clean and your code maintainable.
By understanding how states work and how transitions animate those states, you can build modern, engaging interfaces with minimal effort.
Want to keep learning?
Sign up on our platform or join our WhatsApp channel here to get more hands-on guides like this, delivered regularly.
See you in the next blog. Until then, keep practicing and happy learning!
3 Reactions
0 Bookmarks