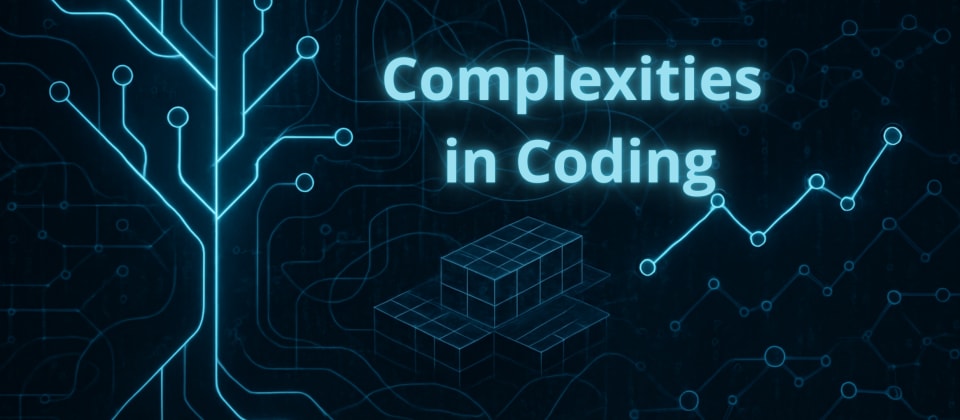
Complexities in Coding
Topic Name: Complexities(Time and Space)
Overview
- What do you understand about complexities?
- Why complexity in a code is important?
- Different type of complexitites(Space and Time).
- Types, examples and representation of different types of space and time complexities
Introduction
When you dive into the world of programming and algorithms, two terms you'll frequently encounter are time complexity and space complexity. These concepts are crucial for evaluating the efficiency of algorithms, which is essential for writing optimized and effective code. But what do they really mean, and how can you understand them easily? Let's break it down!
What is Time Complexity?
Time complexity measures how the runtime of an algorithm increases as the size of the input grows. It gives you an idea of the algorithm's performance and efficiency. It's usually expressed using Big O notation, which provides an upper bound on the time an algorithm will take to run.
Common Time Complexities
1. O(1) - Constant Time
-
The algorithm runs in constant time regardless of the input size.
-
Example: Accessing an element in an array by index.
2. O(log n) - Logarithmic Time
-
The runtime grows logarithmically with the input size.
-
Example: Binary search in a sorted array.
3. O(n) - Linear Time
-
The runtime grows linearly with the input size.
-
Example: Iterating through an array.
4. O(n log n) - Log-Linear Time
-
Common in efficient sorting algorithms.
-
Example: Merge sort, quick sort.
5. O(n^2) - Quadratic Time
-
The runtime grows quadratically with the input size.
-
Example: Nested loops, such as in bubble sort.
6. O(2^n) - Exponential Time
-
The runtime doubles with each additional element in the input.
-
Example: Recursive algorithms for the Fibonacci sequence.
7. O(n!) - Factorial Time
-
The runtime grows factorially with the input size.
-
Example: Permutations, traveling salesman problem.
Visualizing Time Complexity
Here's a simple table to visualize these complexities:
Time Complexity | Example Algorithm | Growth Rate |
---|---|---|
O(1) | Array index access | Constant |
O(log n) | Binary search | Logarithmic |
O(n) | Linear search | Linear |
O(n log n) | Merge sort | Log-linear |
O(n^2) | Bubble sort | Quadratic |
O(2^n) | Fibonacci recursion | Exponential |
O(n!) | Permutation generation | Factorial |
What is Space Complexity?
Space complexity measures the amount of memory an algorithm needs to run as the input size grows. Like time complexity, it's also expressed using Big O notation. Space complexity considers both the fixed part and the variable part (which depends on the input size).
Common Space Complexities
1. O(1) - Constant Space
-
The algorithm uses a fixed amount of memory.
-
Example: Simple arithmetic operations.
2. O(n) - Linear Space
-
The memory usage grows linearly with the input size.
-
Example: Storing elements in an array or list.
3. O(log n) - Logarithmic Space
-
The memory usage grows logarithmically with the input size.
-
Example: Algorithms using divide-and-conquer strategy, like binary search.
4. O(n^2) - Quadratic Space
-
The memory usage grows quadratically with the input size.
-
Example: Dynamic programming algorithms storing intermediate results.
Visualizing Space Complexity
Here's a simple table to visualize these complexities:
Space Complexity | Example Algorithm | Growth Rate |
---|---|---|
O(1) | Simple Arithmetic Operations | Constant |
O(log n) | Binary search | Logarithmic |
O(n) | Taking Array elements | Linear |
O(n^2) | Dynamic Programming Algorithm | Quadratic |
Why Complexity Matters
Understanding time and space complexity is crucial because:
-
Efficiency: Helps you write code that runs faster and uses less memory.
-
Scalability: Ensures your algorithm can handle large inputs without degrading performance.
= Optimization: Guides you in improving your code by identifying bottlenecks.
Conclusion
Time and space complexity are fundamental concepts in computer science that help you understand and optimize the performance of your algorithms. By learning to recognize different complexities and their implications, you can write more efficient and effective code. Remember, the goal is to find a balance between time and space efficiency for your specific problem.
Hope it helps
7 Reactions
2 Bookmarks