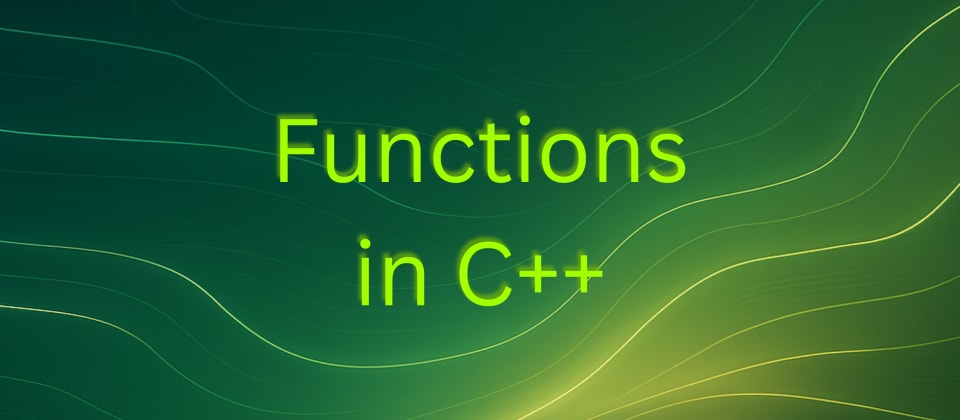
Functions in C++
Overview
- What is a function?
- Need of functions.
- Structure of a function.
- Types of function.
- Parameters of a function.
What is a function?
A function is a set of statements that takes input,does some specific operations, and produces output. The main use of using a function is to avoid writing the same code again and again for different inputs.
In simple terms , function is a block of code that runs only when called.
Syntax
Example
// C++ Program to demonstrate working of a function
#include <iostream>
using namespace std;
// Following function that takes two parameters 'x' and 'y'
// as input and returns max of two input numbers
int max_no(int x, int y)
{
if (x > y)
return x;
else
return y;
}
// main function that doesn't receive any parameter and
// returns integer
int main()
{
int a = 10, b = 20;
// Calling above function to find max of 'a' and 'b'
int m = max_no(a, b);
cout << "m is " << m;
return 0;
}
Output
m is 20
Time complexity: O(1)
Space complexity: O(1)
Why do we need Functions?
- Functions help us in reducing code redundancy. If functionality is performed at multiple places in software, then rather than writing the same code, again and again, we create a function and call it everywhere. This also helps in maintenance as we have to make changes in only one place if we make changes to the functionality in future.
- Functions make code modular. Consider a big file having many lines of code. It becomes really simple to read and use the code, if the code is divided into functions.
- Functions provide abstraction. For example, we can use library functions without worrying about their internal work.
Structure of a function
The structure of a function has three levels
-
Prototype: This is the declaration of fucntion with its return type and its parameters along with their data type.
-
Definition: This is the block of code where specific operations perform on the arguments.
-
Calling: This is where the function is called to perform operations on specific inputs.
Note: You can do prototype and definition together
Example
#include<bits/stdc++.h>
using namespace std;
int max_no(int a,int b); //function prototype of int return type
int main(){
int a=10,b=20,m;
m=max_no(a,b); //calling of a function with inputs a and b
cout<<"maximum number is "<<m;
return 0;}
int max_no(int a, int b) //function definition
{
if (a>b)
return a;
else
return b;}
Types of Functions
There are mainly two types of function in C++
- User Defined Function
- Library Function
User Defined Function
User-defined functions are user/customer-defined blocks of code specially customized to reduce the complexity of big programs. They are also commonly known as “tailor-made functions” which are built only to satisfy the condition in which the user is facing issues meanwhile reducing the complexity of the whole program.
Library Function
Library functions are also called “built-in Functions“. These functions are part of a compiler package that is already defined and consists of a special function with special and different meanings. Built-in Function gives us an edge as we can directly use them without defining them whereas in the user-defined function we have to declare and define a function before using them.
For Example: sqrt(), max(), strcat(),strlen() etc.
Parameters of a Function
- The parameters passed to the function are called actual parameters. For example, in the program below, 10 and 20 are actual parameters.
The parameters received by the function are called formal parameters. For example, in the below program a and b are formal parameters.
#include<bits/stdc++.h>
using namespace std;
int max_no(int a, int b){ //formal parameters
if(a>b)
return a;
else
return b;
}
int main(){
int a=10,b=20;
m=max_no(a,b); //actual parameters
cout<<"maximum number is: "<<m;
return 0;
}
Conclusion
In this blog, we understood the basic concepts of functions, structure of the function, formal and actual parameters, user-defined and library functions, need of functions along with proper images and examples.
Thank you for reading
Happy Coding!!
7 Reactions
1 Bookmarks