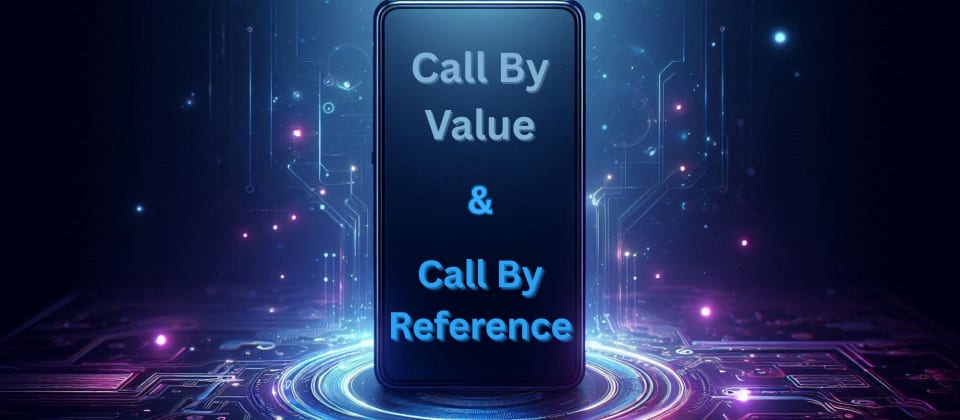
Call by Value & Call by Reference
Overview
- Definition
- Uses
- Limitations
- Difference
- Examples with outputs
Introduction
In C++ programming language, we can pass arguments to functions in different ways mainly by Call by Value and Call by Reference method. They differ by the type of values passed through them as parameters.
Quick Recall:
The actual parameters also known as arguments are the parameters that are passed into the function when we make a function call whereas formal parameters are the parameters that we see in the function or method definition i.e. the parameters received by the function.
Call by Value
The call by value method allows you to copy the actual parameter to a formal parameter. In this case, if we change the formal parameter then the actual parameter doesn’t change.
In other words, the value of the parameter is duplicated into the memory location designated for the function’s parameter. Consequently, two memory locations now hold the same value. C++ uses call by value method by default.
Use of Call by Value
- When you do not want to change the actual parameters of the function.
- When you want to make copies of the data instead of the actual data.
- When space is not an issue.
- Usually, when you do not deal with recursion or backtracking.
Limitations of Call by Value
- Data passed is then stored in temporary memory
- Can’t operate over the actual data rather need to work on temporary copy made of the data, because of which changes made in the data in the function is not reflected in main function.
- Memory Space required while using string ,array , vector, etc can be huge.
- Tackling Backtracking and recursion can be complex using call by values.
Example
//in cpp
#include <bits/stdc++.h>
using namespace std;
void swap_no(int x, int y)
{
int t = x;
x = y;
y = t;
cout << "After Swapping in function x: " << x
<< ", y: " << y << endl;
}
int main()
{
int x = 1, y = 2;
cout << "Before Swapping: ";
cout << "x: " << x << ", y: " << y << endl;
swap_no(x, y);
cout << "After Swapping: ";
cout << "x: " << x << ", y: " << y << endl;
return 0;
}
Output
Before Swapping:
x: 1, y: 2
After Swapping in function x:2, y:1
After Swapping:
x: 1, y: 2
As you can see in the output, the numbers are swapped inside the function but the original numbers are still not swapped.
Call by Reference
In call by reference method of parameter passing, the address of the actual parameters is passed to the function as the formal parameters. In C++, we use pointers or &(address of) sign to achieve call-by-reference.
- Both the actual and formal parameters refer to the same locations.
- Any changes made inside the function are actually reflected in the actual parameters of the caller.
Use of Call by Reference
-
Call by reference can be more efficient than call by value, especially for large data structures like arrays, vectors, and objects. It avoids making a copy of the argument, which can save both time and memory.
-
Call by reference allows functions to modify the actual argument values. This can be useful when you need a function to change the state of the passed variables.
-
By using references, you can return multiple values from a function without needing to use complex data structures. You can pass multiple variables to the function, and it can modify each one.
-
Ensures that changes made in the function persist after the function call, leading to more predictable and consistent behavior when dealing with complex data manipulations.
Limitations of Call by Reference
-
Since the function can modify the original argument, there's a risk of unintended side effects if the function inadvertently alters the data. This can lead to bugs that are hard to trace.
-
Call by reference can make the code less clear and harder to understand. It's not always immediately obvious whether a function will modify its arguments, especially if the function signature isn't well documented.
-
For those new to the concept, call by reference might not be as intuitive as call by value, leading to misunderstandings and errors.
-
While call by reference can save time and memory, it can also introduce overhead due to the additional memory indirection involved. This might be negligible in most cases, but it's something to consider in performance-critical applications.
Example
//in cpp
#include <bits/stdc++.h>
using namespace std;
void swap_no(int &a, int &b) {
int temp = a;
a = b;
b = temp;
}
int main() {
int x = 10, y = 20;
cout << "Before swap: x = " << x << ", y = " << y << endl;
swap_no(x, y);
cout << "After swap: x = " << x << ", y = " << y << endl;
return 0;
}
Output
Before swap: x = 10, y = 20
After swap: x = 10, y = 10
As you can see, this time the original value get swapped.
Difference between Call by Reference and Call by Value
Aspect | Call By Value | Call By Reference |
---|---|---|
Definition | While calling a function, we pass the values of variables to it. Such functions are known as "Call By Values". | While calling a function, instead of passing the values of variables, we pass the address of variables (location of variables) to the function known as "Call By References". |
Argument Handling | In this method, the value of each variable in the calling function is copied into corresponding dummy variables of the called function. | In this method, the address of actual variables in the calling function is copied into the dummy variables of the called function. |
Effect on Original Variables | With this method, the changes made to the dummy variables in the called function have no effect on the values of actual variables in the calling function. | With this method, using addresses we would have access to the actual variables and hence we would be able to manipulate them. |
Modification of Original Variables | In call-by-values, we cannot alter the values of actual variables through function calls. | In call by reference, we can alter the values of variables through function calls. |
Technique | Values of variables are passed by the Simple technique. | Pointer variables are necessary to define to store the address values of variables. |
Preferred Use Case | This method is preferred when we have to pass some small values that should not change. | This method is preferred when we have to pass a large amount of data to the function. |
Safety | Call by value is considered safer as original data is preserved. | Call by reference is risky as it allows direct modification in original data. |
Conclusion
In conclusion, Call by Value means passing values as copies to the function, so that the original data is preserved and any changes made inside the function are not reflected in the original data, whereas Call by Reference means passing references to the memory locations of variables, hence changes made inside the function are directly modified in the original values. The choice between the two completely depends on the particular requirements and considerations of a program.
Thanks for reading!!
Happy coding..
6 Reactions
1 Bookmarks