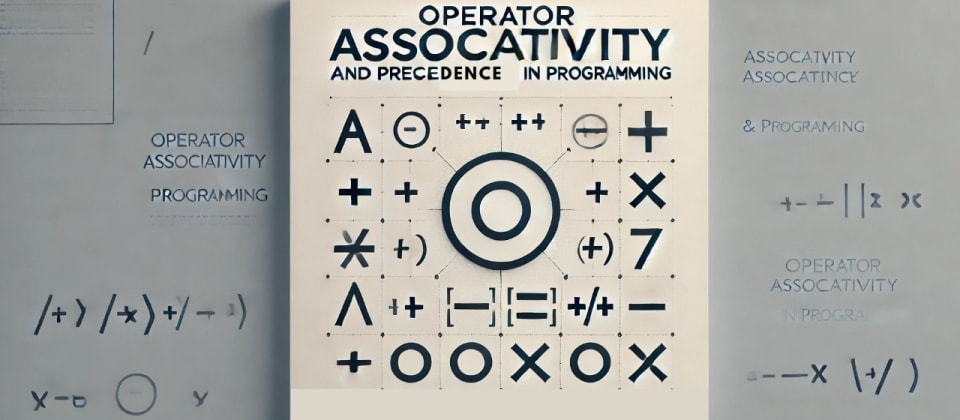
Precedence and Associativity
Introduction
Hey Coders! Have you ever wondered why some expressions in code evaluate differently than you initially expected? This often comes down to two fundamental concepts: Precedence and Associativity. In this blog, we'll see what associativity and precedence are, their significance, and how they affect the way your code runs.
Overview
- What is Precedence?
- What is Associativity?
- Real life examples of Precedence and Associativity.
- Why Precedence and Associativity matters?
- Precedence and Associativity Table for operators.
- Conclusion.
Precedence
- Let's take real world example for precedence:
Example 1: Making a Sandwich
1.High Precedence (Multiplication, Division): First you have to take essential ingredients like bread and butter.
2.Low Precedence (Addition, Subtraction): Adding toppings like tomatoes and lettuce.
So, if you think your sandwich as bread * butter + tomatoes
, you first put the butter on the bread and then add the tomatoes.
- In technical terms:
Precedence determines the order in which operators are evaluated in an expression. When multiple operators are present, higher-precedence operators are evaluated before lower-precedence ones. Think of it as a set of rules that tell the compiler or interpreter which operations to perform first to ensure the correct execution of the program.
Example 2:
int result = 3 + 4 * 2;
Here, multiplication(*
) has a higher precedence than addition(+
). Thus, 4 * 2
is evaluated first, resulting in 3 + 8
, which equals 11
.
Why Precedence Matters?
Precedence rules help to remove confusion in expressions. For example, in complex mathematical equations, these rules ensure that operations are performed in the correct order, leading to the intended results. Without precedence rules, the same expression could yield different outcomes depending on the order of operations.
Precedence Table:
Precedence Level | Operator | Description |
---|---|---|
1 (Highest) | () |
Parentheses |
2 | ++ , -- |
Postfix increment/decrement |
3 | + , - |
Unary plus and minus |
4 | * , / , % |
Multiplication, division, modulo |
5 | + , - |
Addition, subtraction |
6 | << , >> |
Bitwise shift |
7 | < , <= , > , >= |
Relational operators |
8 | == , != |
Equality operators |
9 | & |
Bitwise AND |
10 | ^ |
Bitwise XOR |
11 | | |
Bitwise OR |
12 | && |
Logical AND |
13 | || |
Logical OR |
14 | ?: |
Ternary conditional |
15 (Lowest) | = , += , -= etc. |
Assignment |
Associativity
- Let's start by taking real world example for associativity:
Example 1: Writing a Message in Different Languages
1.Left to Right (Hindi, English): When writing something in Hindi or English, we always write from left to right.
2.Right to Left (Urdu): While writing the same message in Urdu, we write it from right to left.
So, in both the cases, we are writing message in languages(both Hindi and Urdu has same precedence), but both of them have different rules of writing it.
- In technical terms:
Associativity defines the direction in which an expression is evaluated when two operators of the same precedence level appear. Operators can be left-associative or right-associative. Left-associative operators are evaluated from left to right, whereas right-associative operators are evaluated from right to left.
Example 2:
int result = 10 - 4 - 2;
Here, subtraction(-
) is left-associative, Thus, 10 - 4
is evaluated first, followed by 6 - 2
, resulting in 4
.
Why Associativity Matters?
Associativity resolves conflicts when multiple operators of the same precedence level are present. It ensures that expressions are evaluated in a predictable manner.
Associativity Table:
Operator Type | Operators | Associativity Direction |
---|---|---|
Unary operators | + , - , ++ , -- |
Right-to-left |
Multiplication Division Modulo | * , / , % |
Left-to-right |
Addition Subtraction | + , - |
Left-to-right |
Shift operators | << , >> |
Left-to-right |
Relational | < , <= , > , >= |
Left-to-right |
Equality | == , != |
Left-to-right |
Bitwise AND | & |
Left-to-right |
Bitwise XOR | ^ |
Left-to-right |
Bitwise OR | | |
Left-to-right |
Logical AND | && |
Left-to-right |
Logical OR | || |
Left-to-right |
Conditional (ternary) | ?: |
Right-to-left |
Assignment | = , += , -= etc. |
Right-to-left |
Conclusion
Understanding associativity and precedence is crucial for writing clear and correct code. These concepts help programmers to predict how their code will execute, avoid common errors, and debug more effectively. Remember, when in doubt, use parentheses to explicitly specify the order of operations in your expressions, ensuring that your code behaves as intended.
Happy Coding!
7 Reactions
0 Bookmarks