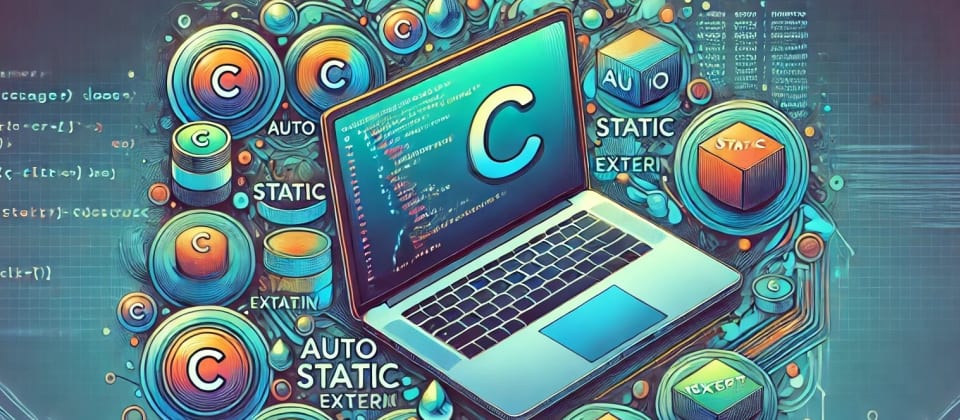
Storage Classes
Hey Coders!
Programming in C is like being the director of a play — every variable in your program is an actor, and storage classes determine how each actor behaves on stage. Who gets the spotlight? Who works behind the scenes? Who remembers their lines even after the curtain falls? Let’s explore storage classes with fun, real-life comparisons and examples to make these concepts memorable!
Table of Content:
- Different storage classes
- auto
- register
- static
- extern
- Traits of storage classes
- defualt value
- scope
- lifetime
- linkage
- memory location
- typical usage
- Examples of different storage classes
What are Storage Classes in C
Storage classes in C define the following key traits of a variable:
-
Storage duration: How long does the variable stay in memory?
-
Scope: Where can the variable be accessed in the program?
-
Linkage: Can the variable be shared across different files?
-
Memory location: Where is the variable stored (e.g., stack or CPU register)?
In C, there are four main storage classes:
- auto
- register
- static
- extern
1.auto
: The Temporary Worker
Think of auto
variables as temporary workers. They show up for the task (a function or block), do their job, and leave when the task is done. These variables are the default for local variables, so you rarely need to use the auto
keyword explicitly.
Real-Life Analogy:
Imagine you hire a helper to clean your house for a day. Once the job is done, they leave, and you can’t call on them again.
Attributes:
-
Storage duration: Temporary, exists only during the execution of the block or function.
-
Scope: Local to the block or function.
-
Linkage: None, as it cannot be accessed outside the function or block.
-
Memory location: Stored in the stack.
Example:
#include <stdio.h>
void example() {
auto int x = 5; // Explicit usage of auto
int y = 10; // Implicitly auto
printf("x: %d, y: %d\n", x, y);
}
int main() {
example();
return 0;
}
Output:
x: 5, y: 10
Here, x
and y
are created when the function runs and destroyed when it ends. They’re temporary and only exist inside the function.
2. register
: The Speed Enthusiast
register
variables are like sprinters in a race — they need to be fast. These variables are stored in CPU registers (if possible) instead of regular memory, making access quicker. Use them for frequently used variables, such as counters in loops.
Real-Life Analogy:
Imagine storing your most-used tools in your pocket instead of a toolbox. You can grab them instantly when needed.
Attributes:
-
Storage duration: Temporary, exists only during the execution of the block or function.
-
Scope: Local to the block or function.
-
Linkage: None, as it cannot be accessed outside the function or block.
-
Memory location: Stored in CPU registers (if possible).
Example:
#include <stdio.h>
void example() {
register int i;
for (i = 0; i < 5; i++) {
printf("Fast Counter: %d\n", i);
}
}
int main() {
example();
return 0;
}
Output:
Fast Counter: 0
Fast Counter: 1
Fast Counter: 2
Fast Counter: 3
Fast Counter: 4
Here, i
is suggested to be stored in a CPU register for faster access during the loop.
3. static
: The Memory Keeper
The static
storage class is like a loyal assistant who remembers everything you’ve told them, even after a meeting ends. Static variables retain their values between function calls, making them perfect for keeping track of state information.
Real-Life Analogy:
Think of a whiteboard in your office/class. You can write on it during a meeting/lecture, and even after the meeting/lecture ends, the notes stay there for future reference.
Attributes:
-
Storage duration: Exists for the entire duration of the program.
-
Scope: Local to the block (for local static variables) or file (for file-level static variables).
-
Linkage: None for local static variables; internal linkage for file-level static variables.
-
Memory location: Stored in the data segment of memory.
Example:
#include <stdio.h>
void counter() {
static int count = 0; // Keeps its value between calls
count++;
printf("Count: %d\n", count);
}
int main() {
counter();
counter();
counter();
return 0;
}
Output:
Count: 1
Count: 2
Count: 3
Here, count
is initialized only once and retains its value across function calls.
Here, count is initialized only once and retains its value across function calls.
4. extern: The Global Connector
The extern
storage class is the ultimate team player, allowing variables to be shared between different files. It declares a variable without defining it, signaling that the actual definition is elsewhere.
Real-Life Analogy:
Think of a community bulletin board. You post a note in one neighborhood, and people from other neighborhoods can see and use it.
Attributes:
-
Storage duration: Exists for the entire duration of the program.
-
Scope: Global, accessible across files.
-
Linkage: External linkage, allowing it to be shared across files.
-
Memory location: Stored in the data segment of memory.
Example:
File1.c
#include <stdio.h>
int sharedVariable = 42; // Defined here
File2.c
#include <stdio.h>
extern int sharedVariable; // Declared here
int main() {
printf("Shared Variable: %d\n", sharedVariable);
return 0;
}
Merge the two files...by make declaration file as header file File1.h
using .h extension and then use #include "File1.h"
in second file.
Or you can use this command....
gcc file1.c file2.c -o output
./output
Output:
Shared Variable: 42
Comparison Table
Storage Class | Default Value | Scope | Lifetime | Linkage | Memory Location | Typical Usage |
---|---|---|---|---|---|---|
auto |
Garbage value | Local | Within block/function | None | Stack | Implicitly used |
register |
Garbage value | Local | Within block/function | None | CPU registers (if possible) | For fast variables |
static |
0 | Local/File | Entire program execution | None (local), Internal (file) | Data segment | Persistent values |
extern |
0 | Global (files) | Entire program execution | External | Data segment | Shared variables |
When Should You Use Each Storage Class?
-
auto: For most local variables; it’s the default.
-
register: For frequently accessed variables in performance-critical sections.
-
static: To retain state information across function calls or limit the scope of global variables.
-
extern: To share variables across multiple files.
With these storage classes, you can make your C programs efficient and well-organized. Experiment with them and watch how they shape your code’s behavior!
For more amazing content Click Here
Till then Happy Coding!!!
8 Reactions
0 Bookmarks