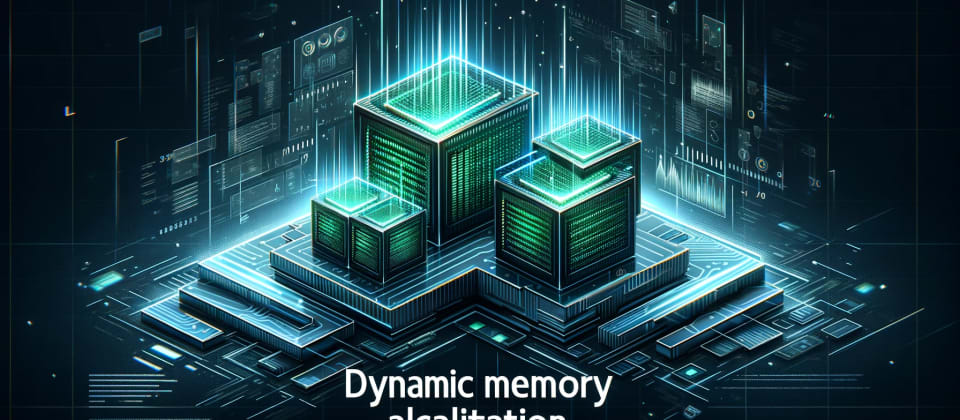
Dynamic Memory Allocation
Dynamic memory allocation means giving your program extra space to store information while it’s running. Think of it like deciding to rent more storage space because you didn’t know earlier how much you’d need.
Overview:
- Introduction
- Types of memory usage
- Static Memory
- Dynamic Memory
- Types of dynamic memory allocation
- malloc()
- calloc()
- realloc()
- free()
- Comparison between malloc() and calloc()
- Common Tips and Mistakes
What is Dynamic Memory Allocation?
Imagine you are packing for a trip. If you know the exact number of items, you can use a fixed-size suitcase. But what if you don’t know? You’d want a flexible suitcase that can expand. That’s what dynamic memory allocation does for programs – it gives them adjustable space.
In C, there are two types of memory usage:
- Fixed Memory (Static): Like a fixed-size suitcase. Once chosen, you can’t change its size.
- Flexible Memory (Dynamic): Like an expandable suitcase. You can decide the size as you go.
This flexible memory comes from a special storage area in your computer called the "heap."
How Does C Handle Dynamic Memory?
C provides simple tools to get, adjust, and free up extra memory. These tools are like commands you give to rent, expand, or return your storage space. These commands are found in the <stdlib.h>
library.
1. malloc()
- Rent Space
malloc()
gives you a block of space. But remember, it doesn’t clean up this space – it could have random stuff left over from before!
int *numbers = (int *)malloc(10 * sizeof(int)); // Rent space for 10 numbers
if (numbers == NULL) {
// If renting fails
}
2. calloc()
- Rent and Clean
calloc()
also gives you a block of space, but it cleans it first, so it starts empty.
int *numbers = (int *)calloc(10, sizeof(int)); // Rent and clean space for 10 numbers
if (numbers == NULL) {
// If renting fails
}
3. realloc()
- Resize Space
realloc()
lets you adjust the size of the space you rented earlier. Need more room? No problem.
numbers = (int *)realloc(numbers, 20 * sizeof(int)); // Expand to fit 20 numbers
if (numbers == NULL) {
// If resizing fails
}
4. free()
- Return Space
free()
is like giving back the storage space when you’re done with it.
free(numbers); // Return the rented space
numbers = NULL; // Avoid accidents by resetting the pointer
Comparison between malloc
and calloc
Feature | malloc() |
calloc() |
---|---|---|
Initialization | Does not initialize memory (contains garbage values). | Initializes memory to zero. |
Parameters | Takes one parameter (size in bytes). | Takes two parameters (number of elements and size of each element). |
Syntax | ptr = (type *)malloc(size); |
ptr = (type *)calloc(num, size); |
Operator Used | Uses multiplication (* ) for total size calculation manually. |
Automatically calculates total size using num * size . |
Speed | Generally faster. | Slightly slower due to initialization. |
Use Case | When initialization is not required. | When memory needs to be zero-initialized. |
Simple Tips to Remember
- Always Check If You Got Space: When renting space, check if it worked. If it didn’t, you’ll get
NULL
, which means there’s no space available.
int *data = (int *)malloc(100 * sizeof(int));
if (data == NULL) {
printf("Could not get space!\n");
}
-
Don’t Forget to Return Space: Always use
free()
when you’re done with the space to avoid wasting memory. -
Start Fresh When Needed: Use
calloc()
if you want your space to be clean (empty) when you get it. -
Be Safe After Returning Space: After using
free()
, set your pointer toNULL
so you don’t accidentally use space that’s no longer there.
free(data);
data = NULL; // Now it’s safe
Avoid Common Mistakes
- Not Returning Space: Forgetting to use
free()
means your program keeps using memory, even when it doesn’t need it. Over time, this could crash your program. - Returning the Same Space Twice: If you use
free()
more than once on the same space, your program might behave unpredictably. - Using Freed Space: After using
free()
, don’t try to access that space. It’s no longer yours.
A Simple Example
Here’s a simple example of how dynamic memory allocation works:
#include <stdio.h>
#include <stdlib.h>
int main() {
int n;
printf("How many numbers do you want to store? ");
scanf("%d", &n);
// Rent space for n numbers
int *numbers = (int *)malloc(n * sizeof(int));
if (numbers == NULL) {
printf("Could not get enough space!\n");
return 1;
}
// Store and print numbers
for (int i = 0; i < n; i++) {
numbers[i] = i + 1;
}
printf("Your numbers: ");
for (int i = 0; i < n; i++) {
printf("%d ", numbers[i]);
}
printf("\n");
// Return the space when done
free(numbers);
return 0;
}
Conclusion:
Dynamic memory allocation is like flexible storage for your program. If you don’t know how much space you’ll need ahead of time, you can rent, resize, and return space as needed. It makes your programs smarter and more adaptable. Just remember to be careful and tidy up when you’re done.
Happy Coding!!!
For more amazing content...Click Here
5 Reactions
0 Bookmarks