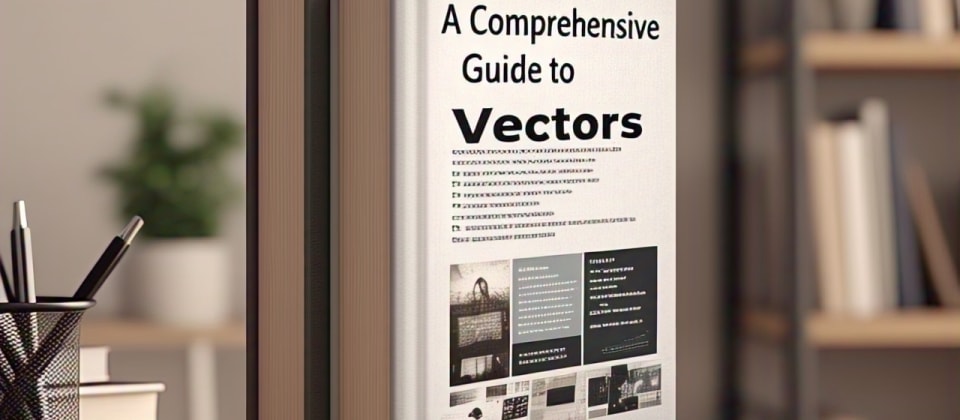
Vectors in C++
Think of vectors as magic backpacks that grow or shrink to fit whatever you throw into them. Whether you're new to C++ or preparing for competitive programming challenges, this guide will show you how to harness the power of vectors—from simple one-dimensional lists to complex multi-dimensional grids. And yes, we’re using the handy #include <bits/stdc++.h>
header to keep things concise and contest-friendly.
Table of Contents
- Why Vectors Rock!!
- One -Dimensional Vectors
- Declaring and Initializing
- Common Method and Operations
- Multi-Dimensional Vectors
- Declaring and Initializing
- Accessing and Modifying Elements
- Performance Considerations
- Iterators and STL: The Dynamic Duo
- Best Practice of Vectors
- Conclusion
1. Why Vectors Rock!!
Imagine having a backpack that automatically expands when you add more items. Vectors work the same way! They are dynamic arrays that handle memory for you, meaning you don't have to worry about allocating or deallocating space manually. Plus, with built-in methods and seamless STL (Standard Libraries) integration, vectors are useful for rapid development and competitive programming.
Tip:In competitive programming and contests, programmers often include #include<bits/stdc++.h>
to access all standard libraries in one go. If working on a project that include large data it is prescribed to use #include<vector.h>
library to use vectors.
2. One-Dimensional Vectors:
Declaring and Initializing:
For simple data storage, one-dimensional vectors are the go-to choice. Here’s how you can declare and initialize them:
#include <bits/stdc++.h>
using namespace std;
int main() {
// Declaration of an empty vector of integers.
vector<int> numbers;
// Initializing with values
vector<int> primes = {2, 3, 5, 7, 11};
// Creating a vector with a predefined size and default value.
vector<int> zeros(10, 0); // 10 elements, each set to 0
return 0;
}
Syntax: vector<data_type>vector_name(initial size,initial or default value);
Note: initial size and value are optional.
Common Methods and Operations:
Below is a table that summarizes many useful methods for one-dimensional vectors:
Method/Operator | Usage | Description |
---|---|---|
push_back() |
numbers.push_back(42); |
Appends an element to the end of the vector. |
pop_back() |
numbers.pop_back(); |
Removes the last element from the vector. |
insert() |
numbers.insert(numbers.begin() + 1, 15); |
Inserts an element at the specified position. |
erase() |
numbers.erase(numbers.begin()); |
Removes an element at the specified position. |
clear() |
numbers.clear(); |
Removes all elements, leaving the vector empty. |
operator[] |
int value = numbers[0]; |
Direct element access (no bounds checking). |
at() |
int value = numbers.at(0); |
Element access with bounds checking. |
front() |
int first = numbers.front(); |
Accesses the first element. |
back() |
int last = numbers.back(); |
Accesses the last element. |
size() |
size_t sz = numbers.size(); |
Returns the current number of elements. |
capacity() |
size_t cap = numbers.capacity(); |
Returns the total allocated memory (element capacity). |
3.Multi-Dimensional Vectors:
When your problems demand grids, matrices, or tables of data, multi-dimensional vectors (most commonly 2D vectors) are your best friend.
Declaring and Initializing :
A two-dimensional vector is essentially a vector of vectors. For instance, to create a 3x3 matrix filled with zeros:
#include <bits/stdc++.h>
using namespace std;
int main() {
// Declare a 3x3 2D vector with all elements set to 0.
vector<vector<int>> matrix(3, vector<int>(3, 0));
// Alternatively, initialize with explicit values:
vector<vector<int>> grid = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
return 0;
}
2D vector is simply called as vector of vectors meaning it is a vector in which each element (row) is a vector of specified data type.
Syntax: vector<vector<data_type>> vector_name(number_of_rows, vector<int>(number_of_columns,initial or default value));
Accessing and Modifying Elements:
Accessing elements in a 2D vector uses two indices—one for the row and one for the column:
#include <bits/stdc++.h>
using namespace std;
int main() {
vector<vector<int>> grid = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
// Accessing the element at the second row, third column:
int value = grid[1][2]; // value is 6
// Modifying an element:
grid[1][2] = 10; // Changes the element 6 to 10
// Traversing a 2D vector with nested loops:
for (int i = 0; i < grid.size(); i++) {
for (int j = 0; j < grid[i].size(); j++) {
cout << grid[i][j] << " ";
}
cout << "\n";
}
return 0;
}
Extra Tips for Multi-Dimensional Vectors:
- Resizing:
Use push_back()
or pop_back()
on the outer vector to add or remove rows.
// Adding a new row to the grid
vector<int> newRow = {10, 11, 12};
grid.push_back(newRow);
- Dynamic Column Modifications:
Each row is a vector, so you can modify columns individually using push_back()
, insert()
, or erase()
.
// Insert an element in the first row at the second position
grid[0].insert(grid[0].begin() + 1, 99);
4. Performance Considerations:
When coding for performance—especially under contest conditions—it's crucial to know the time complexities of vector operations. Here’s a quick summary:
Operation | Time Complexity | Quick Note |
---|---|---|
Access ([] , at() ) |
O(1) | Super-fast, constant time access. |
push_back() |
Approx O(1) | Occasional reallocation may slow things down briefly. |
pop_back() |
O(1) | Instantaneous removal of the last element. |
Insertion at beginning | O(n) | Requires shifting all elements. |
Erasing at beginning | O(n) | Similar shifting overhead as insertion. |
Size/Empty | O(1) | Quick property checks. |
5. Iterators and STL:The Dynamic Duo:
Iterators are like bookmarks that let you navigate through your vector effortlessly. They are also essential for integrating with STL algorithms.
Traversing with Iterators:
#include <bits/stdc++.h>
using namespace std;
int main() {
vector<int> numbers = {10, 20, 30, 40, 50};
// Traversing using iterators
for (auto it = numbers.begin(); it != numbers.end(); ++it) {
cout << *it << " ";
}
return 0;
}
Note: auto
is a keyword that automatically detects and assigns the data type to the variable.
STL Algorithms:
Are you tired of writing sorting functions to sort your array.If yes, then sorting a vector is just a one-liner with STL:
#include <bits/stdc++.h>
using namespace std;
int main() {
vector<int> numbers = {50, 10, 40, 20, 30};
sort(numbers.begin(), numbers.end());
for (int n : numbers)
cout << n << " ";
return 0;
}
Below is a quick reference table for some common STL algorithms used with vectors:
Algorithm | Header | What It Does |
---|---|---|
sort() |
<algorithm> |
Arranges elements in ascending order. |
reverse() |
<algorithm> |
Reverses the order of elements. |
find() |
<algorithm> |
Searches for a specific element. |
accumulate() |
<numeric> |
Computes the sum of the elements. |
Note: In find()
, you have to also provide value you want to find apart from starting and ending index.
6. Best Practices with Vectors:
Here are some best practices to make your code cleaner and more efficient when using vectors:
- Memory Management:
Use the reserve()
method when you know the approximate size ahead of time. This minimizes the overhead of frequent reallocations.
vector<int> numbers;
numbers.reserve(100); // Pre-allocate memory for 100 elements.
- Safety vs Speed:
Use at()
for bounds-checked access when debugging. For performance-critical sections, operator[]
is faster—but be careful!
- Minimize Copies:
When passing vectors to functions, use references to avoid unnecessary copying.
7. Conclusion:
Vectors are not just another container in C++—they’re a versatile tool that transforms how you manage data. Whether you’re working with a simple list of numbers or a complex matrix, understanding vectors in all their forms is essential for efficient programming and competitive problem-solving. With dynamic resizing, rich functionality, and seamless STL integration , vectors empower you to write cleaner, faster code.
Keep practicing, experiment with different operations, and soon you'll be using vectors with the confidence of a seasoned programmer.
Happy coding, and may your vectors always be dynamic and efficient!
7 Reactions
0 Bookmarks