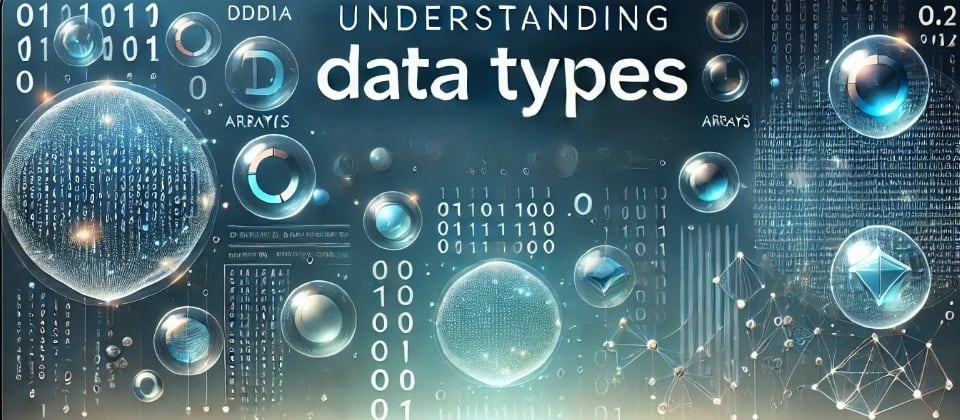
Data Types
Hey Coders! Understanding data types is essential for programming in any language. Data types are the foundation upon which variables are defined, operations are performed, and memory is allocated. They ensure that data is stored efficiently and accurately, providing a way to identify the kind of data we're working with.
In this blog, we'll explore the various data types you'll encounter in programming languages like C, C++. From primitive types like integers and floating-point numbers to more complex composite types like arrays and structures, we'll cover it all. By the end, you'll have a comprehensive understanding of how data types shape the way you code and manage data in different contexts.
Overview
- Primitive Data Types
- int (int,short,long,long long)
- char
- float (float, double, long double)
- bool
- Derived Data Types
- Arrays
- Pointers
- Structures
- Unions
- Enumeration
- Difference between structure and union
- Data Type Conversion
- Implicit Conversion
- Explicit Conversion
Primitive Data Types
Primitive data types are the basic building blocks of data manipulation in programming. They represent simple values and are defined by the language itself.
Primitive Data types in C++:
Type | C | C++ | Format Specifier | Description | Size (Bytes) |
---|---|---|---|---|---|
int |
Yes | Yes | %d or %i |
Standard integer | Typically 4 |
short |
Yes | Yes | %hd |
Short integer | Typically 2 |
long |
Yes | Yes | %ld |
Long integer | At least 4 |
long long |
Yes (C99) | Yes | %lld |
Longer integer | Typically 8 |
char |
Yes | Yes | %c |
Single character | 1 |
float |
Yes | Yes | %f |
Single-precision floating-point | Typically 4 |
double |
Yes | Yes | %lf |
Double precision floating-point | Typically 8 |
long double |
Yes | Yes | %Lf |
Extended precision floating-point | More than 8 |
bool |
No | Yes | `Not in C | Boolean value (true/false) | 1 |
Note Points:
-
Size (Bytes): Sizes can vary based on the system architecture and compiler implementations.
-
int: The size of int and other integer types can vary but are typically 4 bytes for int.
-
char: char is signed or unsigned depending on the compiler and system.
-
-
Boolean Types: Before C99, C did not have a standard boolean type directly. C++ introduced bool as part of its standard types.
-
Extended Precision: The size of long double is implementation-dependent and can be greater than 8 bytes.
Derived Data Types
As the name suggests, the Derived data types are user-defined data types that are built from primitive data types to manage more complex structures of data or to provide functionality. By combining or extending the basics, they offer more flexibility and control over how data is stored, accessed and manipulated.
Derived Data Types in C/C++:
1. Arrays:
An array is a collection of elements, all of the same type, stored in contiguous memory locations. The array's length is fixed upon declaration, making it ideal for managing lists of items.
-
Syntax: type arrayName[arraySize];
-
Example:
int numbers[5] = {1, 2, 3, 4, 5};
2. Pointers:
A pointer is a variable that stores the address of another variable. Pointers are crucial for dynamic memory allocation, manipulating arrays, and performing low-level tasks.
-
Syntax: type *pointerName;
-
Example:
int value = 10;
int *ptr = &value; // ptr stores the address of value
Here, &
is address-of operator and *
is dereference operator.
For more info what they do refer to : Operators.
3. Structures:
Structures (struct
) are user-defined data types that group different types of data together. They are practical for defining complex data records.
-
Syntax: struct StructureName {
type1 member1;
type2 member2;
...
}; -
Example:
struct Person {
char name[50];
int age;
};
4. Unions:
Unions are similar to structures but store different data types in the same memory location. They provide a way to store multiple types of data in the same memory space.
-
Syntax: union UnionName {
type1 member1;
type2 member2;
...
}; -
Example:
union Data {
int i;
float f;
char str[20];
};
5. Enumeration:
An enum
(short for "enumeration") is a derived data type in C/C++ that allows the creation of a collection of named integral constants. By using enum, you can assign names to a set of consecutive integer values, making the code more readable and easier to maintain.
-
Syntax: enum enumerationName {
constant1,
constant2,
constant3,
...
}; -
Example:
#include <stdio.h>
// Define an enum for days of week
enum Day {
SUNDAY,
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY
};
int main() {
enum Day today;
today = WEDNESDAY;
printf("Today is day number %d of the week.\n", today);
return 0;
}
Difference between Structure and Union :
Feature | Structure (struct ) |
Union (union ) |
---|---|---|
Definition | A struct is a user-defined data type that groups different data types together under a single name. |
A union is a user-defined data type where all members share the same memory location. |
Memory Allocation | Each member of a struct has its own memory location, resulting in cumulative memory usage. |
All members of a union share the same memory location, the memory allocated is equal to the size of the largest member. |
Member Access | All members can be accessed and used simultaneously. | Only one member can be used at a time, since they share the same memory location. |
Initialization | Multiple members can be initialized at once. | Only the first member can be initialized in the declaration; initializing another member overwrites the previous one. |
Use Case | Used when data of different types need to be grouped together and accessed individually. | Used when different data types are to be stored in the same memory location, but only one type is needed at any time. |
Example | ```c | ```c |
struct { | union { | |
int a; | int a; | |
float b; | float b; | |
char c; | char c; | |
}; | }; | |
Memory Size Example | If int =4 bytes, float =4 bytes, and char =1 byte, the total size of the struct would be 4+4+1=9 bytes (padding may add more). |
The size of a union would be the size of the largest member, which in this case is 4 bytes (either int or float ). |
Example Program | ``` | ``` |
#include <stdio.h> | #include <stdio.h> | |
struct Data { | union Data { | |
int i; | int i; | |
float f; | float f; | |
char str[20]; | char str[20]; | |
}; | }; | |
int main() { | int main() { | |
struct Data data; | union Data data; | |
data.i = 10; | data.i = 10; | |
printf("data.i: %d\n", data.i); | printf("data.i: %d\n", data.i); | |
Data Type Conversion:
Data type conversion in C and C++ involves changing a variable from one data type to another. It can be classified into two main types: Implicit Conversion (also known as type coercion) and Explicit Conversion (also known as type casting).
Implicit Data Type Conversion:
Implicit data type conversion occurs when the compiler automatically converts one data type to another to perform operations seamlessly. This type of conversion is done without any explicit command from the programmer and helps avoid potential data loss or errors in mixed-type expressions.
Importance:
-
Simplifies Code: Reduces the need for explicit casting, making code more readable.
-
Prevents Errors: Avoids potential data loss or type mismatch errors during arithmetic and logical operations.
-
Ensures Compatibility: Allows different data types to interact fluidly, enhancing the versatility of the code.
Types of Implicit Conversions
- Integer Promotion
Integer promotion is a type of implicit conversion where smaller integer types (char
and short
) are promoted to int
. This promotion ensures that integer arithmetic operations are performed on compatible data types, optimizing performance and preventing errors.
#include <stdio.h>
int main() {
char c = 'A'; // 'A' is 65 in ASCII
int i = c; // Implicit conversion from char to int
printf("i: %d\n", i); // Outputs: i: 65
return 0;
}
In this example, the char
variable c
is automatically promoted to an int
when assigned to i
.
- Arithmetic Conversions
When performing arithmetic operations between different data types, the compiler implicitly converts the operands to a common type, often the type with the highest rank to preserve precision.
#include <stdio.h>
int main() {
int i = 10;
double d = 3.14;
double result = i + d; // 'i' is implicitly converted to double
printf("result: %f\n", result); // Outputs: result: 13.140000
return 0;
}
Here, the integer i
is implicitly converted to a double
before the addition to ensure the result is accurate.
- Rank of Types
The rank of types determines the order of conversions during arithmetic operations. C follows a specific hierarchy to decide the common type in mixed-type expressions:
- long double
- double
- float
- unsigned long long
- long long
- unsigned long
- long
- unsigned int
- int
Explicit Data Type Conversion
Explicit type conversion is the process of manually converting a variable from one data type to another using cast operators. This method is used when you need to convert data types that aren’t implicitly convertible or when you want to force a conversion to ensure the program behaves as intended.
Importance:
- Precision Control: Prevents unwanted effects of implicit conversions.
- Avoids Data Loss: Carefully manages data to avoid truncation or rounding errors.
- Ensures Correctness: Enforces specific data types in operations and assignments, maintaining code correctness and readability.
Syntax: (type) expression;
#include <stdio.h>
int main() {
double d = 9.3;
int i = (int)d; // Explicit conversion from double to int (fractional part is truncated)
printf("The integer value is: %d\n", i); // Outputs: The integer value is: 9
return 0;
}
Types of Explicit Conversions:
- Converting Floating-Point to Integer
When converting from a floating-point type (like float
or double
) to an integer, the fractional part is truncated:
#include <stdio.h>
int main() {
double pi = 3.14159;
int truncatedPi = (int)pi; // Converts double to int, truncating the fractional part
printf("Truncated value of pi: %d\n", truncatedPi); // Outputs: Truncated value of pi: 3
return 0;
}
- Converting Integer to Floating-Point
Converting an integer to a floating-point type is straightforward:
#include <stdio.h>
int main() {
int num = 42;
float floatNum = (float)num; // Converts int to float
printf("Float value: %f\n", floatNum); // Outputs: Float value: 42.000000
return 0;
}
Thanks for Reading!
Click Here for more amazing content...
6 Reactions
0 Bookmarks